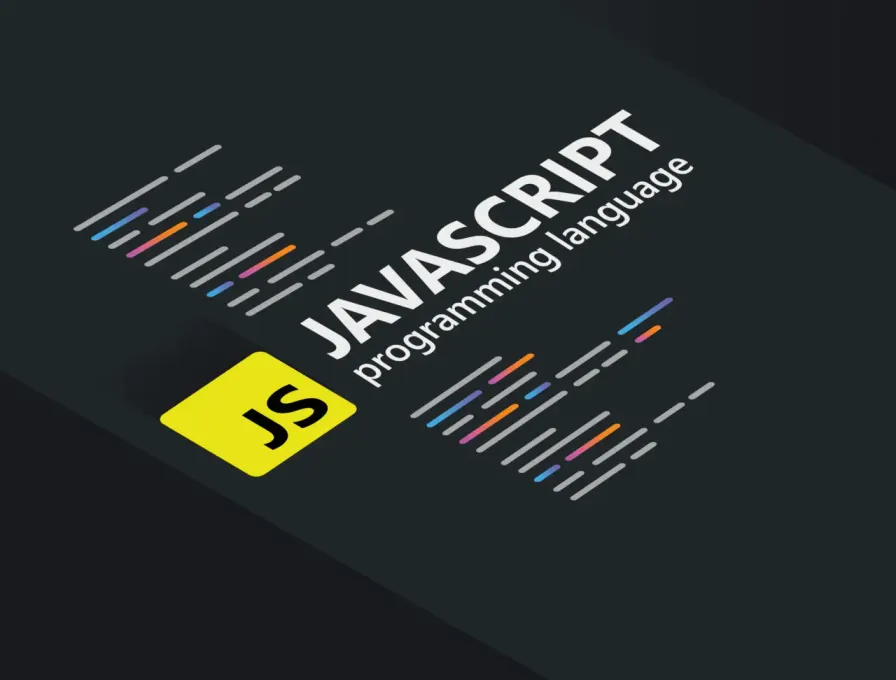
#javascript
Michał Uzdowski
The wild world of JavaScript - One line at a time
Greetings, fellow adventurers! Today, we’re embarking on an epic quest through the magical realm of JavaScript – the language that powers the web and tickles our coding neurons like no other. So strap on your coding capes, grab your trusty keyboards, and let’s dive headfirst into the wonderful world of JavaScript, where the code flows like mead at a Viking feast, and the curly braces are our battle cries. And should you happen to be a newcomer to the lands of web development, may I also present you the Mastering HTML and CSS guide.
A Brief History Lesson
Come, gather ‘round, ye seekers of knowledge, and let me regale you with the epic tale of JavaScript – a saga as rich and varied as the threads of code that weave through its digital tapestry. Our journey begins in the hallowed halls of Netscape Communications Corporation, circa the mid-1990s, where a young programmer by the name of Brendan Eich was tasked with a monumental challenge: to create a scripting language that would bring interactivity to the fledgling World Wide Web.
And so, with a stroke of his coding wand, Eich conjured forth JavaScript – a language that would forever alter the course of the internet’s destiny. But make no mistake, dear reader, for JavaScript’s ascent to web domination was far from assured. In those early days, it was a scrappy underdog, overshadowed by its more established siblings like Java and C++.
But oh, how the tides would turn! As the web grew and evolved, so too did JavaScript, morphing and adapting to meet the ever-changing needs of developers and users alike. With the rise of dynamic web applications and the advent of AJAX (Asynchronous JavaScript and XML), JavaScript emerged as a force to be reckoned with – a versatile language capable of everything from form validation to dynamic content loading.
Yet, JavaScript’s journey was not without its trials and tribulations. From browser inconsistencies to security vulnerabilities, JavaScript faced its fair share of challenges along the way. But with each obstacle overcome, it emerged stronger and more resilient, a testament to the indomitable spirit of its creators and community.
And so, here we stand, in the dawn of a new era – an era where JavaScript reigns supreme as the undisputed king of the web. From humble beginnings in the halls of Netscape to its current status as the backbone of modern web development, JavaScript’s journey is a testament to the power of innovation, collaboration, and sheer determination.
So raise a toast to JavaScript, dear readers, for it is more than just a programming language – it is a symbol of human ingenuity, creativity, and the boundless possibilities of the digital age. And as we continue our own quests for knowledge and mastery, let us never forget the humble origins and heroic journey of JavaScript – the language that changed the web forever.
The Modern Era: ES6 and Beyond
Yes, yes I’ve already mentiond all the young age pains JavaScript was bserving. But wait, there’s more! In the modern era, JavaScript has undergone a glorious transformation with the introduction of ECMAScript 6 (ES6 for short). With ES6, developers gained access to a treasure trove of new features, from arrow functions and template literals to destructuring and the mighty spread operator.
// ES5 function
function add(a, b) {
return a + b;
}
// ES6 arrow function
const add = (a, b) => a + b;
// ---
// ES5 string concatenation
var name = "John";
var greeting = "Hello, " + name + "!";
// ES6 template literal
const name = "John";
const greeting = `Hello, ${name}!`;
// ---
// ES5 object destructuring
var person = { name: "John", age: 30 };
var name = person.name;
var age = person.age;
// ES6 object destructuring
const person = { name: "John", age: 30 };
const { name, age } = person;
// ---
// ES5 array concatenation
var arr1 = [1, 2, 3];
var arr2 = [4, 5, 6];
var combined = arr1.concat(arr2);
// ES6 spread operator
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combined = [...arr1, ...arr2];
But ES6 was just the beginning. With each passing year, new features and improvements are added to the JavaScript ecosystem, making it more powerful, versatile, and downright delightful to work with. And with the rise of frameworks like React, Vue, and Angular, JavaScript’s reign over the web is more secure than ever.
Node.js: Expanding JavaScript’s Horizons
Ah, but there’s even more to the JavaScript saga! With the advent of Node.js, JavaScript transcended its humble origins in the browser and found new life outside the confines of web development. Node.js, a runtime environment built on Chrome’s V8 JavaScript engine, allows developers to run JavaScript on the server-side, opening up a world of possibilities for building scalable, real-time applications, APIs, and even desktop applications.
// Node.js example
const http = require("http");
const server = http.createServer((req, res) => {
res.writeHead(200, { "Content-Type": "text/plain" });
res.end("Hello, world!\n");
});
server.listen(3000, "localhost", () => {
console.log("Server running at http://localhost:3000/");
});
In this Node.js example, we create a simple HTTP server that responds with “Hello, world!” to any incoming requests. With Node.js, JavaScript developers can leverage their existing skills to build powerful server-side applications, tapping into the vast ecosystem of npm packages and libraries to accelerate their development process. So, whether you’re building web servers, command-line tools, or even IoT devices, Node.js has got you covered, proving once and for all that JavaScript is truly a language for all seasons.
Mastering the Fundamentals
Now, let’s talk shop – or rather, let’s talk about the core functions and conventions that make JavaScript the beast that it is. From arrays and objects to loops and conditionals, mastering the fundamentals is key to becoming a JavaScript wizard.
// Behold, the humble array
const adventurers = ["warrior", "mage", "rogue"];
// Looping through the array like a boss
for (let adventurer of adventurers) {
console.log(`The ${adventurer} approaches!`);
}
// Embracing the power of objects
const hero = {
name: "Sir Codalot",
class: "Knight",
level: 42,
};
// Accessing object properties like a true hero
console.log(`${hero.name} is a level ${hero.level} ${hero.class}.`);
But what about conventions, you ask? Fear not, dear reader, for JavaScript has its own set of conventions and best practices to guide you on your quest. From camelCase variable names to meaningful function names and consistent indentation, following these conventions will earn you the respect of your fellow developers and the admiration of your code editor.
Variables and Data Types: The Building Blocks of JavaScript
At the heart of JavaScript lies the concept of variables – containers for storing and manipulating data. But not all variables are created equal! JavaScript boasts a plethora of data types, each with its own unique quirks and characteristics.
-
Primitive Data Types: These are the basic building blocks of JavaScript, including:
Numbers: Integers, floats, and everything in between. Behold, the mighty number type!
Strings: Sequences of characters enclosed in single or double quotes. Let your words weave tales of wonder with the string type!
Booleans: True or false values, perfect for making decisions and branching logic. Embrace the power of true and false with the boolean type!
Undefined: When a variable has been declared but not assigned a value. It’s like a blank canvas, waiting to be filled with meaning.
Null: The absence of a value. It’s the void, the abyss – or maybe just an empty box. -
Complex Data Types: Beyond the realm of primitives lies a world of complexity, where objects and arrays reign supreme.
Objects: Bundles of key-value pairs, allowing you to represent complex data structures with ease. From person objects with name and age properties to more elaborate constructs like DOM elements, objects are the Swiss army knives of JavaScript.const person = { name: "John Doe", age: 30, profession: "Developer", };
Arrays: Ordered collections of elements, perfect for storing lists of data. Whether it’s a list of numbers, strings, or even other arrays, arrays have your back.
const numbers = [1, 2, 3, 4, 5]; const fruits = ["apple", "banana", "orange"];
The Power of Methods: Unleashing JavaScript’s Mightiest Tools
But wait – there’s more! JavaScript isn’t just about storing data; it’s about manipulating it, transforming it, and making it dance to your tune. And that’s where methods come in – the secret sauce that adds flavor to your code and brings it to life.
-
String Methods: Need to manipulate strings? JavaScript has you covered with a treasure trove of string methods, including:
toUpperCase() and toLowerCase(): Transforming strings to uppercase or lowercase, because sometimes you need to SHOUT or whisper.
trim(): Removing whitespace from the beginning and end of a string. Because nobody likes extra spaces – except maybe astronauts.
split(): Breaking a string into an array of substrings based on a delimiter. It’s like slicing a pizza into delicious, bite-sized pieces. -
Array Methods: Arrays are more than just lists – they’re dynamic, versatile, and packed with useful methods:
push() and pop(): Adding and removing elements from the end of an array. It’s like a conveyor belt for your data.
shift() and unshift(): Adding and removing elements from the beginning of an array. Think of it as rearranging the seating chart at a dinner party.
forEach(): Iterating over an array and executing a function for each element. It’s like having a personal assistant to handle your data chores.
Navigating the Maze: Mastering JavaScript Conditions and Loops
As you traverse the treacherous terrain of JavaScript, you’ll inevitably encounter the need to make decisions, repeat tasks, and navigate through different paths in your code. Dread not, for conditions and loops are here to guide you through the maze of logic and steer your code in the right direction. Let me give you a little taste of each.
Conditional Statements
-
If-Else Statements: The stalwart guardians of decision-making in JavaScript, if-else statements allow you to execute different blocks of code based on a condition. Whether it’s checking if a variable is equal to a certain value or evaluating a complex expression, if-else statements are your trusty companions on the journey.
const age = 18; if (age >= 18) { console.log("You are an adult!"); } else { console.log("You are not yet an adult."); }
-
Switch Statements: When you have multiple conditions to evaluate, switch statements come to the rescue. With their elegant syntax and ability to handle multiple cases, switch statements offer a cleaner alternative to long chains of if-else statements.
const day = "Monday"; switch (day) { case "Monday": console.log("It's Monday, time to conquer the week!"); break; case "Friday": console.log("TGIF! Time to unwind and relax."); break; default: console.log("Just another day of the week."); }
-
Ternary Operator: For those times when you need to make a quick decision in a single line of code, the ternary operator is your go-to tool. With its concise syntax, the ternary operator allows you to express conditional logic in a compact and readable manner.
const isRaining = true; const weatherMessage = isRaining ? "Remember to bring an umbrella!" : "Enjoy the sunshine!"; console.log(weatherMessage);
Loops
-
For Loops: As you journey through the vast expanse of JavaScript, you’ll often find yourself needing to perform a task multiple times. Worry not, for the for loop is here to save the day! With its elegant syntax and ability to iterate over arrays, objects, and more, the for loop is your faithful companion in the battle against repetition.
for (let i = 0; i < 5; i++) { console.log(`Iteration ${i + 1}`); }
-
While Loops: When you need to perform a task repeatedly until a certain condition is met, the while loop is your steadfast ally. With its simple syntax and flexibility, the while loop allows you to execute code as long as a condition remains true.
let count = 0; while (count < 5) { console.log(`Count: ${count}`); count++; }
So, fear not the twists and turns of JavaScript conditions and loops, for with if-else statements, switch statements, for loops, and while loops by your side, you’ll navigate the maze of logic with ease and emerge victorious on the other side. Onward, brave coder, and may your code always follow the right path!
Embracing TypeScript: Elevating JavaScript to New Heights
Ah, but what’s this? As you venture deeper into the realm of JavaScript, you may stumble upon a powerful ally known as TypeScript. TypeScript is not just another JavaScript library or framework; it’s a superset of JavaScript that adds static typing, interfaces, and other advanced features to the language. This means you can catch errors before they happen, write more robust and maintainable code, and unleash the full potential of JavaScript in ways you never thought possible.
Consider the following example:
interface Person {
name: string;
age: number;
profession: string;
}
function greet(person: Person): string {
return `Hello, ${person.name}! You are ${person.age} years old and work as a ${person.profession}.`;
}
const john: Person = {
name: "John Doe",
age: 30,
profession: "Developer",
};
console.log(greet(john)); // Output: Hello, John Doe! You are 30 years old and work as a Developer.
In this TypeScript code snippet, we define an interface called Person, which describes the structure of a person object with name, age, and profession properties. We then define a greet function that takes a Person object as its parameter and returns a greeting message using the properties of the person object. Finally, we create a john object that conforms to the Person interface and pass it to the greet function, resulting in a friendly greeting tailored to John’s details.
So, dear adventurer, as you continue your quest for JavaScript mastery, consider delving into the world of TypeScript – for it may just be the key to unlocking even greater wonders on your coding journey.
Where to Learn and Level Up
Now that you’ve glimpsed the inner workings of JavaScript’s fundamental concepts and powerful methods, you might be wondering: where do I go from here? Stay strong, dear reader, for the path to JavaScript mastery is paved with countless resources and opportunities for learning and growth.
- Online Courses: Platforms like YouTube, Udemy, Coursera, and freeCodeCamp offer a plethora of JavaScript courses for all skill levels, from beginner to advanced.
- Documentation: Dive into the official documentation provided by Mozilla Developer Network (MDN) to explore JavaScript’s intricacies in detail.
- Books: From classics like “Eloquent JavaScript” to modern masterpieces like “You Don’t Know JS” there’s no shortage of JavaScript books to guide you on your journey.
- Community: Join online communities like Stack Overflow, Reddit’s r/javascript, and GitHub to connect with fellow JavaScript enthusiasts, ask questions, and share knowledge.
Conclusion: The Adventure Continues
And there you have it, brave adventurers – a whirlwind tour of the wild world of JavaScript. From its humble beginnings to its modern-day reign over the web, JavaScript continues to captivate and inspire developers around the globe. So grab your swords (or rather, your keyboards), and let’s embark on this coding adventure together. Happy coding, and may your curly braces always be balanced!