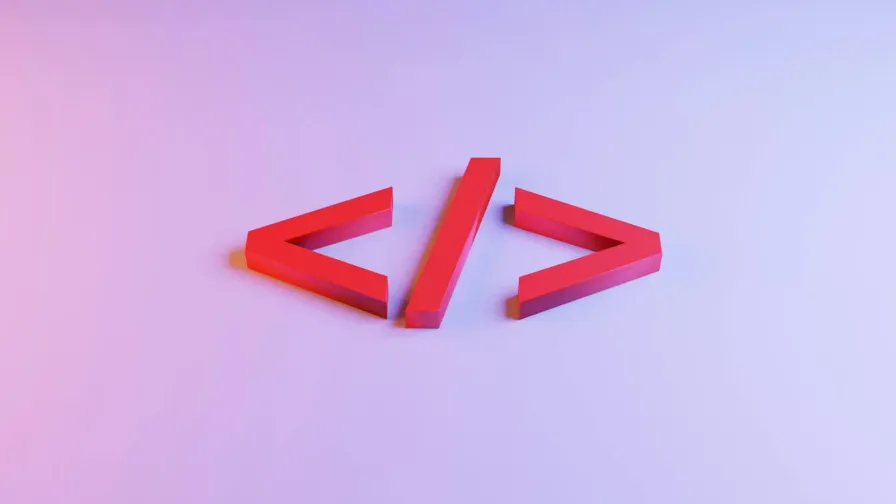
#webdev
Michał Uzdowski
Welcome to web development
Welcome to the world of web development! In this article, we’ll embark on a journey to explore the fundamental concepts and technologies that power the web. Whether you’re a complete beginner or looking to expand your knowledge, this guide will lay a solid foundation for your web development journey.
What is Web Development?
Web development encompasses the creation and maintenance of websites and web applications. It involves a combination of programming languages, frameworks, and tools to build interactive and dynamic experiences for users across the internet.
Frontend vs Backend Development
Frontend Development
Frontend development focuses on the user interface and user experience of a website or web application. It involves writing code that runs in the user’s web browser and determines how the content is presented and interacted with. Key technologies used in frontend development include:
- HTML (Hypertext Markup Language): Defines the structure and content of web pages.
- CSS (Cascading Style Sheets): Styles the HTML elements to control their appearance and layout.
- JavaScript: Adds interactivity and dynamic behavior to web pages.
Definitely check Mastering HTML and CSS and The Wild World of JavaScript - One Line at a Time to find out how to start your web development journey.
Backend Development
Backend development, on the other hand, deals with the server-side logic and database management of a web application. It involves writing code that runs on the server and handles tasks such as processing user requests, accessing databases, and generating dynamic content. Common technologies used in backend development include:
- Node.js: A runtime environment for executing JavaScript code on the server.
- Express.js: A web application framework for building RESTful APIs and handling HTTP requests.
- Databases: Such as MongoDB (NoSQL) or MySQL (SQL) for storing and managing data.
Javascript Frameworks
JavaScript frameworks are collections of JavaScript code libraries that provide developers with pre-written code to use for routine programming features and tasks—essentially a tool to manage and enhance the complexity of using JavaScript on a website or application. These frameworks are designed to support the development of web applications including web services, web resources, and web APIs. Frameworks aim to alleviate the overhead associated with common activities performed in web development.
JavaScript frameworks can differ significantly from one another, each offering its own unique blend of functionalities, which can include templating, reactive interfaces, or comprehensive data binding to synchronize your UI and data sources seamlessly. Some popular examples include React, Angular, and Vue.js for frontend and Express.js or Fastify for backend. Each framework has its strengths and use cases, which are explored in more depth in our feature article, An Extensive Guide to Popular JavaScript Frameworks. This guide will help you understand which framework might be the best fit for your project, and how to get started with it.
Introduction to HTML, CSS, and JavaScript
HTML
HTML is the standard markup language for creating the structure and content of web pages. In the provided example, HTML is used to define the layout of the webpage, including elements such as headers, navigation bars, sections, and footers.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My First Website</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
<main>
<section>
<h2>About Me</h2>
<p>I'm a web developer passionate about creating awesome websites.</p>
</section>
</main>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
CSS
CSS is used to style the HTML elements, controlling their appearance and layout. In the provided example, CSS is applied to set the fonts, colors, margins, padding, and background of various elements, ensuring a visually appealing and consistent design.
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
header {
background-color: #333;
color: #fff;
padding: 20px;
text-align: center;
}
nav {
background-color: #666;
padding: 10px;
}
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
}
nav ul li {
display: inline;
margin-right: 10px;
}
nav ul li a {
color: #fff;
text-decoration: none;
}
main {
padding: 20px;
}
footer {
background-color: #333;
color: #fff;
padding: 10px;
text-align: center;
}
JavaScript
JavaScript adds interactivity and dynamic behavior to web pages. In the provided example, JavaScript is included to demonstrate the use of event listeners, such as waiting for the DOMContentLoaded event before executing code, allowing for dynamic manipulation of webpage content.
// Add interactivity when DOM content is loaded
document.addEventListener("DOMContentLoaded", function () {
console.log("DOM loaded");
// Write JavaScript code here
});
Servers and APIs
Server
Server is a computer program or device that provides functionality for other programs or devices, known as clients. In the context of web development, server typically refers to a computer program that serves web pages to clients over the internet. Servers handle requests from clients and respond with the requested resources, such as HTML documents, images, or data.
APIs (Application Programming Interfaces)
An API is a set of rules and protocols that allows different software applications to communicate with each other. In web development, APIs are commonly used to define the interactions between client-side and server-side components of a web application. They provide a standardized way for clients to request and receive data from a server.
Example
Suppose we have a web application that allows users to retrieve information about books from a server. The server exposes an API with endpoints for fetching book data.
Server-side (Node.js with Express.js)
// server.js
const express = require("express");
const app = express();
// Define route to handle GET requests for retrieving book data
app.get("/api/books", (req, res) => {
// Simulated database query to fetch book data
const books = [
{ id: 1, title: "The Great Gatsby", author: "F. Scott Fitzgerald" },
{ id: 2, title: "To Kill a Mockingbird", author: "Harper Lee" },
{ id: 3, title: "1984", author: "George Orwell" },
];
// Return the book data as JSON response
res.json(books);
});
// Start the server
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Client-side (JavaScript with Fetch API)
// client.js
// Fetch book data from the server
fetch("/api/books")
.then((response) => {
// Check if the request was successful
if (!response.ok) {
throw new Error("Failed to fetch book data");
}
// Parse the JSON response
return response.json();
})
.then((books) => {
// Display the book data on the webpage
books.forEach((book) => {
console.log(`Title: ${book.title}, Author: ${book.author}`);
});
})
.catch((error) => {
console.error(error.message);
});
In this example
The server exposes an API endpoint ‘/api/books’ to retrieve book data. The client-side JavaScript code uses the Fetch API to make a GET request to the ‘/api/books’ endpoint. Upon receiving the response from the server, the client-side code processes the book data and displays it on the webpage or performs further actions as needed. This illustrates how servers and APIs work together to facilitate communication between the client and server components of a web application.
Conclusion
In this article, we’ve scratched the surface of web development by exploring its basic concepts and technologies. HTML, CSS, and JavaScript form the backbone of frontend development, while backend development involves server-side programming and database management. Tackling the task with the right tool is making the job much easier, so we’ve got you covered in Comprehensive Toolbox for Web Development article. Stay tuned for more in-depth tutorials and articles as we dive deeper into the exciting world of web development. Happy coding!