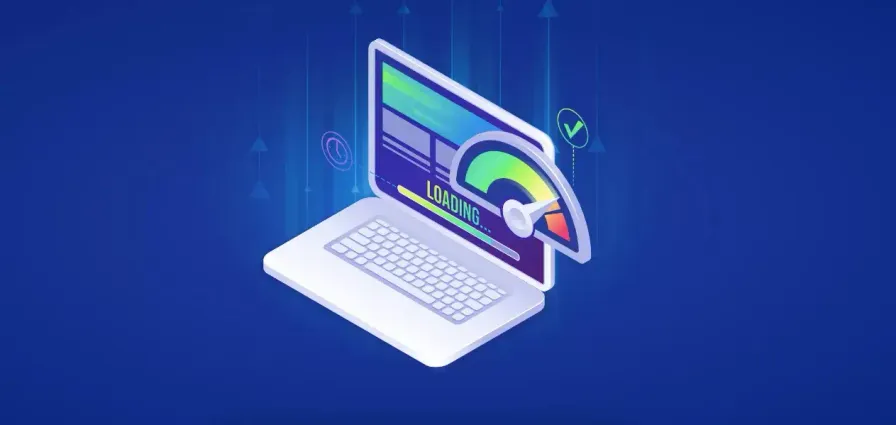
#optimization
Michał Uzdowski
Exploring web performance optimization techniques: Speed is everything
Welcome back to Binary Brain, where we take the complexities of web development, turn them inside out, sprinkle in some humor, and serve it up fresh. Today, we’re tackling a topic that can make or break your web application: Web Performance Optimization.
Let’s be honest — no one enjoys waiting for a slow website to load. In the world of the internet, patience is thinner than a single pixel line, and if your site takes more than three seconds to load, your visitors will bounce faster than a kid off a sugar high. That’s why optimizing your web app’s performance is essential.
But where do you start? Should you shrink every image down to a potato or rip out all your JavaScript? Don’t worry, we’re here to break down the best Web Performance Optimization techniques in a way that’s both fun and digestible. And, of course, we’ll throw in some real-life examples to help you along the way. So, buckle up, because we’re about to turbocharge your site!
Why Web Performance Optimization Matters
Before we dive into the how-to, let’s talk about why optimizing your web app’s performance is so important.
- User Experience: A faster website leads to a better user experience. The smoother and quicker your site loads, the more likely users are to stick around and explore.
- SEO Boost: Google loves fast websites. Site speed is a ranking factor, and the faster your site, the better chance it has of climbing the search engine ladder.
- Conversion Rates: Speed can directly impact your conversion rates. Studies have shown that a one-second delay in page load time can result in a 7% reduction in conversions. In the world of e-commerce, that’s like leaving money on the table!
Think of your website like a pizza delivery. If it’s hot and arrives quickly, your customers are thrilled. But if it’s late and cold, they’re going to be hangry — and probably order from someone else next time.
Key Web Performance Optimization Techniques
Now that we’ve established why speed is king, let’s dive into the techniques you can use to make sure your website loads like a sports car on the autobahn.
Lazy Loading: Don’t Load What You Don’t Need (Yet)
Lazy loading is a strategy where images, videos, and other heavy assets are loaded only when they’re about to enter the user’s viewport. This way, you don’t load everything at once, reducing initial load times.
Example: Let’s say your webpage has 20 images, but only the first 5 are visible above the fold. With lazy loading, you load just those first 5, and the rest load as the user scrolls.
How to Implement It:
In HTML5, you can enable lazy loading on images with a simple attribute:
<img src="cat-picture.jpg" alt="Adorable Cat" loading="lazy" />
This is natively supported by most modern browsers, and it’s probably the easiest performance upgrade you can make.
Lazy loading is like hiring a waiter who only brings you food when you’re ready for it, instead of dumping 20 dishes on your table all at once. Because who wants that much food in their face all at once?
Minification: Shrink That Code!
Minification is the process of removing all unnecessary characters from your CSS, JavaScript, and HTML files, like whitespace, comments, and line breaks. This reduces the file size and allows browsers to download and execute your code faster.
Example: Here’s what minification looks like in JavaScript:
Before minification:
function greet(name) {
console.log("Hello, " + name);
}
After minification:
function greet(n) {
console.log("Hello, " + n);
}
By cutting out spaces and unnecessary characters, we’ve reduced the file size while keeping the same functionality.
How to Minify:
There are several tools available to help you minify your code:
- CSS Minification: Use tools like CSSNano or CleanCSS.
- JavaScript Minification: Tools like UglifyJS or Terser can help minify your JavaScript.
If you’re using build tools like Webpack or Gulp, minification can be automated as part of your build process.
Minification is like packing for a trip and squeezing all your clothes into a vacuum-sealed bag. Sure, it’s the same stuff, but now it fits in a much smaller suitcase.
Image Compression: The Secret to Lightweight Visuals
Images are often the biggest culprits behind slow load times. Uncompressed images can eat up precious bandwidth and slow down your website’s performance. Image compression is the process of reducing the file size of your images without significantly sacrificing quality.
Example: Suppose you have a 1MB image on your homepage. Compressing it can reduce the file size to around 200KB or less, drastically cutting down the load time.
How to Compress Images:
- Use tools like TinyPNG or ImageOptim to compress images without losing quality.
- Use the correct image format: Use JPEG for photos, PNG for transparent images, and WebP (which provides better compression) if you want a modern format supported by most browsers.
If you’re building a responsive site, also consider serving different image sizes based on the user’s device.
<img
src="large-image.jpg"
alt="Large Image"
srcset="small-image.jpg 500w, medium-image.jpg 1000w, large-image.jpg 2000w"
/>
Compressing images is like packing your clothes tighter in a suitcase — same clothes, but they take up less space in the bag. Plus, your website won’t feel like it’s carrying the weight of a full wardrobe.
Caching: Don’t Load Things Twice
Caching is like giving your browser a memory. When a user visits your site, the browser stores certain resources (like images, scripts, and stylesheets) so that the next time the user visits, it doesn’t need to download them again. This results in faster load times for repeat visitors.
How to Implement Caching:
- Use HTTP headers like
Cache-Control
to tell the browser how long to store certain files.
Cache-Control: max-age=31536000
This directive tells the browser to cache the resource for 1 year (31536000 seconds).
- Use service workers for more advanced caching techniques, especially for Progressive Web Apps (PWAs). Service workers can cache entire pages and allow users to access them even when they’re offline. Learn more about service workers and PWA in our article on The Rise of Progressive Web Apps (PWA) in 2024: The Future of Web Development?.
Caching is like leaving a cookie jar out on the table. Every time you want a cookie, you don’t need to bake a fresh batch — you just grab one from the jar.
Reduce HTTP Requests: Less is More
Each time your browser requests a file (an image, script, or CSS file), it makes an HTTP request to the server. The more requests, the slower your page loads. By reducing the number of HTTP requests, you can significantly speed up your site.
How to Reduce HTTP Requests:
- Combine CSS and JavaScript files: Instead of loading multiple CSS or JavaScript files, combine them into a single file. This reduces the number of requests the browser has to make.
- Use CSS sprites: If you have multiple small images (like icons), use a CSS sprite to combine them into one image and then use CSS to display only the part of the image you need.
Example: A sprite sheet for icons might look like this:
.icon {
width: 32px;
height: 32px;
background-image: url("icons-sprite.png");
}
.icon-facebook {
background-position: 0 0;
}
.icon-twitter {
background-position: -32px 0;
}
Now you have one image instead of several, cutting down on those pesky HTTP requests.
Reducing HTTP requests is like running errands. Would you rather make one trip to the grocery store and grab everything at once, or drive back and forth for each item? Thought so.
Enable Gzip Compression: Zip It Up!
Gzip is a compression method that reduces the size of your files (HTML, CSS, JavaScript) before sending them to the browser. Smaller files mean faster downloads, and faster downloads mean happier users.
How to Enable Gzip Compression:
Most web servers (like Apache or Nginx) support Gzip compression, and enabling it is usually just a matter of adding a few lines to your configuration file.
For Nginx:
gzip on;
gzip_types text/plain application/javascript text/css;
For Apache:
AddOutputFilterByType DEFLATE text/html text/plain text/css application/javascript
Gzip is like vacuum-sealing your clothes before shipping them. It shrinks everything down so it fits in a smaller package and travels faster.
Tools to Test Your Web Performance
You’ve implemented all these performance tricks, but how do you know if they’ve actually worked?
Luckily, there are plenty of tools to help you test and monitor your web performance:
- Google PageSpeed Insights : Gives you a performance score and suggestions on how to improve your site.
- GTmetrix: Analyzes your page load time and gives detailed reports on what’s slowing you down.
- WebPageTest: Offers in-depth performance analysis, including waterfall charts and video comparisons.
These tools are like personal trainers for your website. They tell you where you’re flabby (slow) and give you exercises (optimizations) to trim the fat.
Conclusion
In the fast-paced world of web development, performance optimization is no longer just a nice-to-have — it’s essential. From lazy loading images to compressing files and caching resources, these techniques will help you create lightning-fast web applications that keep users engaged and coming back for more.
Remember, optimizing your web performance isn’t a one-time task. Keep testing, refining, and updating your site to stay ahead of the curve. And as always, here at Binary Brain, we keep it fun, keep it real, and keep you coding like a pro.
So, go forth, speed up that website, and watch as users flock to your lightning-fast app like moths to a beautifully optimized flame! 🚀