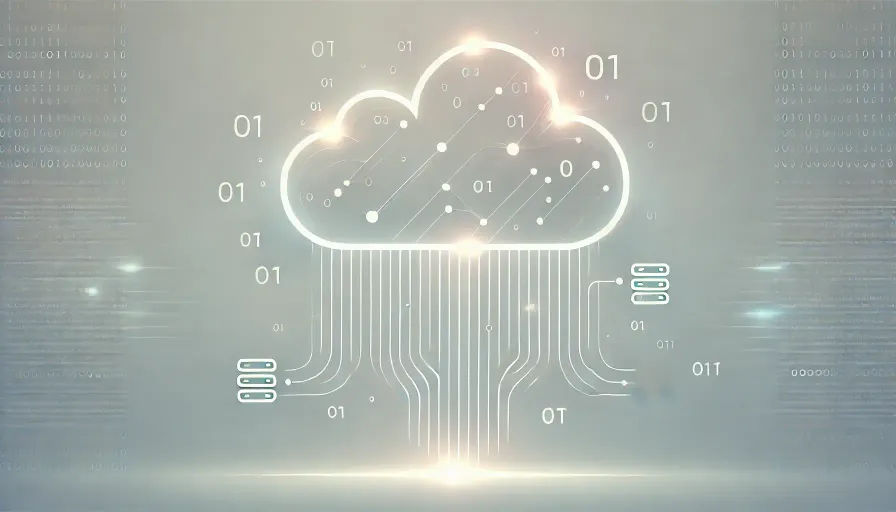
#serverless
Michał Uzdowski
Exploring Serverless Computing: Revolutionizing backend development
Welcome back to Binary Brain, where we navigate the intricate world of technology with a sense of humor and a compass pointing toward simplicity. Today, we’re diving into the magical world of Serverless Computing — the mystical land where servers exist, but you don’t have to manage them. It’s like running a restaurant where someone else does all the cooking, cleaning, and restocking. You just take the credit (and maybe a hefty tip).
Serverless computing is a hot topic in backend development, and for good reason. It promises scalability, lower costs, and freedom from managing infrastructure. So, grab your favorite snack (or serverless meal?), and let’s explore how serverless computing is revolutionizing backend development!
What is Serverless Computing?
Let’s start by clearing up a common misconception: Serverless doesn’t mean there are no servers. There are still servers involved; you just don’t have to worry about them. Your cloud provider (like AWS, Azure, or Google) takes care of the servers, scaling, patching, and all the dirty work. You, as the developer, focus solely on writing code.
Imagine you’re a pilot. Traditional backend development is like flying a plane — you have to worry about fuel, weather, and technical issues. Serverless computing? That’s like being flown around in a private jet. You’re still in the air, but you don’t have to touch a single button.
Popular Serverless Services
Before we dive into the code, let’s take a look at the main players in the serverless arena.
AWS Lambda
AWS Lambda is Amazon’s serverless computing service. You write your code, define a trigger (like an HTTP request or a database event), and Lambda takes care of the rest. It automatically scales to handle incoming requests and only charges you for the compute time your function uses — down to the millisecond!
Lambda is like having a personal assistant that only shows up when you need them and charges by the minute. Efficiency at its finest.
Azure Functions
Microsoft’s Azure Functions are the cool kid on the block for serverless computing. They support multiple programming languages (like C#, JavaScript, Python, and more) and integrate smoothly with the entire Azure ecosystem.
Azure Functions are like that one friend who’s always there to help, no matter what problem you’re facing. Need to send an email, process data, or handle an HTTP request? They’ve got your back.
Google Cloud Functions
Google Cloud Functions lets you run your code in response to events from Google services or any other HTTP-based requests. Like Lambda and Azure Functions, it handles scaling, deployment, and infrastructure, letting you focus on writing code.
Google Cloud Functions is the tech equivalent of a self-driving car. You set the destination (trigger your code), and it handles the rest. You can just sit back and watch the magic happen.
How Does Serverless Work?
Let’s break down how serverless computing actually works behind the scenes:
-
You Write Code: Instead of managing a full application stack (with servers, databases, etc.), you write small, self-contained functions that do specific tasks.
-
Set a Trigger: You set up triggers, like an HTTP request, file upload, or database event, that tell the cloud provider when to execute your function.
-
The Cloud Provider Handles Scaling: Your function will be executed whenever the trigger occurs, and the cloud provider automatically scales your function based on demand. If you get 10 requests, your function runs 10 times. If you get 10,000 requests, your function runs 10,000 times — without you having to lift a finger.
-
You Get Billed Based on Usage: Unlike traditional server setups, where you pay for uptime (whether the server is doing something or not), serverless charges you only for the compute time your function uses. It’s like a pay-as-you-go phone plan for your code.
Benefits of Serverless Computing
So why should you consider using serverless computing for your next backend project? Let’s explore some of the key benefits.
Cost Efficiency
You only pay for the compute time you use, down to the millisecond. Traditional servers charge you for uptime, but with serverless, if your function doesn’t run, you don’t pay. It’s ideal for apps with fluctuating traffic or intermittent workloads.
Paying for traditional servers is like owning a car — you’re paying for it even when it’s sitting in the garage. Serverless is like using a ride-share app — you pay only when you’re actually on the road.
Automatic Scaling
No more panic attacks when your app goes viral, and your server can’t handle the traffic. Serverless computing automatically scales to handle any number of requests, so your app can go from 10 users to 10 million without breaking a sweat.
Imagine throwing a party where extra chefs, bartenders, and cleaning staff magically appear whenever more guests arrive. That’s serverless scaling!
Reduced Maintenance
No more worrying about server patches, security updates, or scaling issues. The cloud provider takes care of all the infrastructure headaches, so you can focus on writing code and developing features.
Traditional backend development is like maintaining an aging car. You’ve got to change the oil, rotate the tires, and pray it doesn’t break down. Serverless is like having a brand-new car that maintains itself. You just drive!
Faster Development
Because serverless functions are small and focused on specific tasks, development is faster and more modular. You can build, test, and deploy individual functions without worrying about the entire application.
Traditional development is like building a house from scratch. Serverless? It’s like IKEA furniture — modular, quick, and (mostly) painless to assemble.
Use Cases for Serverless Computing
So, where does serverless computing really shine? Let’s take a look at some common use cases where it makes perfect sense.
Event-Driven Applications
Serverless functions are perfect for handling event-driven tasks like file uploads, database updates, or user actions. For example, you could trigger a serverless function every time a user uploads a file, automatically resizing and storing images in a cloud storage bucket.
REST APIs
Serverless computing is ideal for building APIs. You can create individual functions for each API endpoint (GET, POST, PUT, DELETE), and the cloud provider will scale them independently based on usage.
Code Example (AWS Lambda Function as an API):
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify({ message: "Hello from your Lambda API!" }),
};
return response;
};
With services like AWS API Gateway, you can map HTTP requests to your Lambda functions and serve an entire REST API with minimal setup.
Scheduled Tasks
Need to run tasks on a schedule? Serverless functions can be triggered by time-based events (like cron jobs). Whether it’s generating reports, cleaning up databases, or sending out automated emails, serverless handles it with ease.
Real-Time Data Processing
Serverless functions can handle real-time data processing, such as analyzing data streams, monitoring IoT devices, or processing user interactions in web apps. For example, you could use Google Cloud Functions to analyze data in real time as it streams from IoT sensors.
Serverless Computing in Action: Building a Simple API
Let’s build a simple API using AWS Lambda. We’ll create a Lambda function that returns a list of items when triggered by an HTTP GET request.
Step 1: Create the Lambda Function
In your AWS Console, go to Lambda and create a new function.
exports.handler = async (event) => {
const items = [
{ id: 1, name: "Serverless Pizza" },
{ id: 2, name: "Serverless Burger" },
{ id: 3, name: "Serverless Salad" },
];
const response = {
statusCode: 200,
body: JSON.stringify(items),
};
return response;
};
This simple function returns a list of “serverless” items as a JSON response.
Step 2: Set Up API Gateway
To make the function accessible via HTTP, go to API Gateway and create a new REST API.
- Add a GET method for the
/items
endpoint. - Link this method to your Lambda function.
Step 3: Test the API
Once everything is set up, test your API by sending a GET request to your /items
endpoint. You should receive a list of serverless items in the response. Congratulations, you’ve built a serverless API!
Challenges of Serverless Computing
While serverless computing is fantastic, it’s not without its challenges. Let’s dive into some of the major pitfalls you might encounter.
Cold Starts
When a serverless function hasn’t been used in a while, the cloud provider may take a few seconds to “warm up” the function, causing a delay. This is known as a “cold start.” It’s like waking up in the morning — there’s a little lag before you’re fully functional.
Vendor Lock-In
Relying heavily on a specific cloud provider’s serverless platform can make it difficult to migrate your application to another provider. It’s like signing an exclusive deal with one restaurant — you might love their food, but if you ever want to try something new, it could be tricky.
Debugging
Debugging serverless functions can be more challenging than debugging traditional applications. Because your functions are executed in a cloud environment, it can be harder to reproduce issues locally. However, tools like AWS CloudWatch and Google Stackdriver can help with monitoring and logging.
Unexpected Bills
One of the most significant risks of serverless computing is the potential for massive, unexpected bills. Because serverless platforms charge based on the number of executions, if your app is exploited or misconfigured, you could end up with an enormous bill for services you didn’t anticipate. For example:
DDoS Attacks: If an attacker floods your app with requests, your serverless functions will scale to handle them — and your bill will scale right along with it.
Unintended Loops: Poorly designed code could result in infinite loops or excessive retries, leading to thousands of function invocations.
Security Exploits: If your app isn’t properly secured, attackers could exploit vulnerabilities, causing unintended or malicious function executions.
It’s like leaving your phone on international roaming and binge-watching Netflix. You’ll enjoy it for a while, but the bill that comes afterward will haunt your dreams.
To avoid this, always implement security measures like rate-limiting, input validation, and proper monitoring to catch unusual activity before it spirals out of control. Setting budget alerts with your cloud provider can also help mitigate any surprise costs.
Conclusion
Serverless computing is revolutionizing backend development by making it faster, more cost-effective, and easier to scale. Whether you’re building APIs, processing data, or automating tasks, serverless offers a powerful and flexible solution that allows you to focus on what you do best: writing code.
So, are you ready to go serverless and let the cloud handle the heavy lifting? Give it a try — you might just find that it’s the perfect solution for your next project. And as always, here at Binary Brain, we’ll be here to guide you through the journey, keeping things fun and informative. Happy coding!