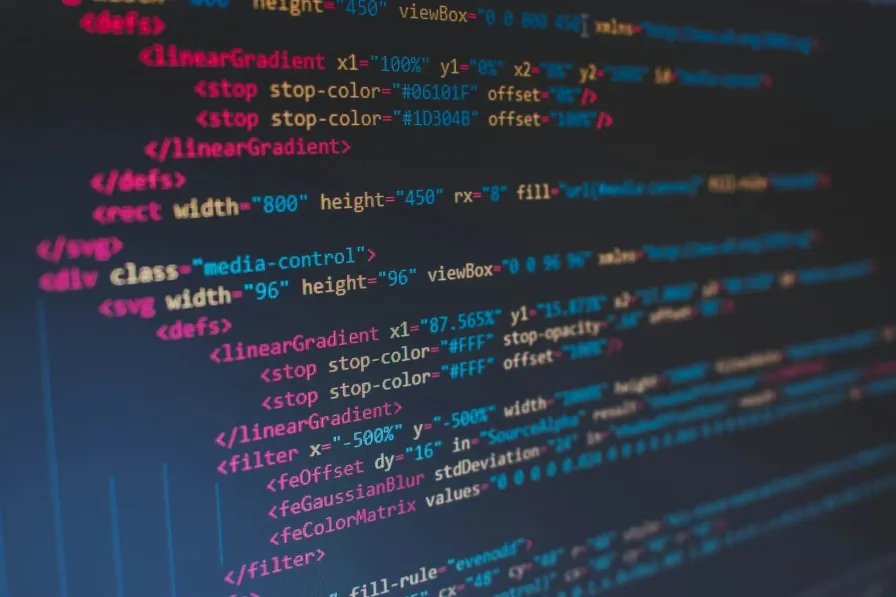
#webdev
Michał Uzdowski
Mastering HTML and CSS
Ahoy, fellow web wanderers! Prepare to embark on a journey through the mystical realms of HTML and CSS, where pixels become poetry and divs dance in harmony. In this epic saga, we’ll delve deep into the art of crafting stunning web pages, uncovering tips, tricks, and a hearty dose of humor along the way. So buckle up, grab your favorite coding snack (may we suggest the classic combo of chips and caffeine?), and let’s set sail on this coding adventure together!
The Fundamentals: HTML & CSS 101
Before we hoist the sails and set off into the unknown, let’s first anchor ourselves in the basics of HTML and CSS. HTML (HyperText Markup Language) lays the foundation for every web page, providing structure and semantics to your content. Think of it as the skeleton of your website – defining headings, paragraphs, images, and more.
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My Awesome Website</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
<section>
<h2>About Us</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit...</p>
</section>
<footer>
<p>© 2024 My Awesome Website. All rights reserved.</p>
</footer>
</body>
</html>
CSS (Cascading Style Sheets), on the other hand, is the magician that adds flair and personality to your HTML elements. With CSS, you can sprinkle colors, fonts, layouts, and animations onto your web pages, transforming them from bland to breathtaking.
/* styles.css */
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
}
header {
background-color: #333;
color: #fff;
text-align: center;
padding: 20px 0;
}
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
}
nav li {
display: inline;
margin-right: 20px;
}
nav a {
text-decoration: none;
color: #333;
}
section {
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
footer {
text-align: center;
padding: 20px 0;
background-color: #333;
color: #fff;
}
Tips and Tricks for HTML & CSS Mastery
-
Embrace Semantic HTML
Don’t just throw
<div>s
around like confetti. Use semantic HTML elements like<header>, <nav>, <section>
, and<footer>
to provide meaning and structure to your content. Not only does it improve accessibility and SEO, but it also makes your code easier to understand. -
Stay Organized with CSS
Keep your CSS organized and maintainable by using meaningful class names, grouping related styles together, and leveraging CSS preprocessors like Sass or Less. Remember, a tidy stylesheet is a happy stylesheet!
-
Responsive Design is Key
With the plethora of devices accessing the web today, it’s essential to make your websites responsive. Use media queries and flexbox / grid layouts to ensure your content looks great on all screen sizes. Your users will thank you, and so will their smartphones.
-
Keep it DRY (Don’t Repeat Yourself)
Avoid duplicating code by using CSS inheritance, mixins, and variables. This not only reduces redundancy but also makes your code easier to maintain and update. Plus, you’ll feel like a coding wizard every time you refactor your stylesheets.
-
Experiment and Have Fun
The web is your playground, so don’t be afraid to experiment with new CSS techniques and HTML features. Want to create a website that looks like a vintage postcard? Go for it! Feeling inspired to build a tribute to your favorite ’90s sitcom? Why not! The only limit is your imagination (and maybe browser compatibility).
More Examples, More Fun!
Let’s spice things up with a few more code examples to tickle your coding taste buds:
Fancy Buttons with CSS Transitions
<!-- html -->
<button class="fancy-btn">Click Me!</button>
Ahoy, matey! Behold, the crown jewel of our webpage – the mighty button, ready to be clicked, tapped, or mashed with reckless abandon. It’s the digital equivalent of a treasure chest, just waiting for intrepid adventurers to discover its secrets.
/* css */
.fancy-btn {
background-color: #4caf50;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
transition-duration: 0.4s;
cursor: pointer;
}
.fancy-btn:hover {
background-color: #45a049;
color: white;
}
In the land of CSS, we’re dressing up our button with all the flair of a pirate’s wardrobe. The background-color property sets the stage for our button’s grand entrance, while padding adds a plush cushion for weary clickers. With transition-duration, we’re giving our button a touch of drama – think of it as the slow-motion scene in an action movie, but with fewer explosions and more color changes. And when the user hovers over our button, it undergoes a magical transformation, like Cinderella at the ball, but with less glass slippers and more stylish color shifts. Ah, the wonders of CSS – where every element is a star in its own right!
Responsive Image Gallery with CSS Grid
<!-- html -->
<div class="gallery">
<img src="image1.jpg" alt="Image 1" />
<img src="image2.jpg" alt="Image 2" />
<img src="image3.jpg" alt="Image 3" />
</div>
In this HTML snippet, we’ve got a posh gallery div with an eclectic mix of images, ready to dazzle your visitors. It’s like hosting a swanky art exhibition, but without the stuffy dress code. The <img>
tags are the star attractions, each promising a visual feast for the eyes.
/* css */
.gallery {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 10px;
}
.gallery img {
width: 100%;
height: auto;
}
Now, in the realm of CSS, we’re styling our gallery with the finesse of a fashion designer at Paris Fashion Week. With the display: grid property, we’re orchestrating a pixel-perfect ballet of images, ensuring they dance gracefully across the screen. The grid-template-columns rule is like the conductor, guiding the layout with the precision of a seasoned maestro. And let’s not forget the gap property – it’s the breathing space between images, preventing them from getting too cozy and causing pixelated drama.
External Resources for Further Learning
Ready to dive deeper into the world of HTML and CSS? Check out these fantastic resources:
HTML
CSS
Playground for practice
So there you have it, fellow coders – a comprehensive guide to mastering HTML and CSS, sprinkled with humor, creativity, and a touch of whimsy. Remember, the web is your canvas, and with HTML and CSS as your paintbrushes, there’s no limit to what you can create. So go forth, craft beautiful websites, and don’t forget to have fun along the way. Happy coding!