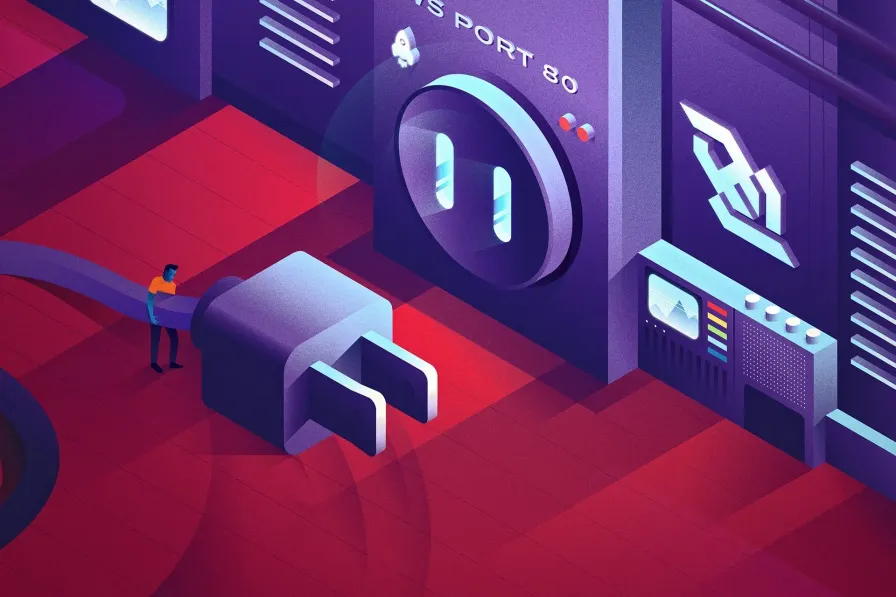
#websockets
Michał Uzdowski
WebSockets: Real-time communication for modern web applications
Welcome back to Binary Brain, where we take you on a rollercoaster ride through the thrilling world of web development. Today, we’re diving into the realm of real-time communication with WebSockets. If you’ve ever wanted to build a chat app, a live feed, or anything that updates faster than your favorite sports ticker, WebSockets are your new best friend. So, grab your favorite beverage, and let’s explore how to bring your web applications into the real-time world!
What Are WebSockets?
Before we get our hands dirty with code, let’s take a moment to understand what WebSockets actually are.
WebSockets are a communication protocol that provides full-duplex (two-way) communication channels over a single TCP connection. Unlike traditional HTTP requests, where the client makes a request and the server responds (and then the connection is closed), WebSockets keep the connection open, allowing for real-time data transfer between the client and server.
Think of HTTP as a polite conversation at a formal dinner party. You say something, the other person responds, and then there’s a pause before the next exchange. WebSockets, on the other hand, are like an intense, fast-paced debate where everyone talks at once — constantly, rapidly, and without waiting for formal turn-taking.
Why Use WebSockets?
Real-time Data
In the modern web, users expect data to be updated instantly — whether it’s stock prices, chat messages, or game scores.
WebSockets enable real-time data updates by keeping an open connection between the client and server, allowing information to flow in both directions without delay.
Imagine you’re watching a live sports game. HTTP is like getting score updates by refreshing your browser every few seconds. WebSockets are like watching the game on TV — you get the updates as they happen, without lifting a finger.
Reduced Latency
HTTP’s request-response cycle introduces latency, as the connection has to be re-established with every request.
WebSockets reduce latency by maintaining a persistent connection, so data can be sent and received immediately without the overhead of reopening a connection.
If HTTP is like having to knock on your neighbor’s door every time you want to borrow some sugar, WebSockets are like having a walkie-talkie — you can chat instantly without all the door-knocking hassle.
Efficient Communication
WebSockets are more efficient for continuous communication, as they eliminate the need for HTTP headers, reducing the size of each message.
By using WebSockets, you can send smaller, more frequent updates without clogging up the network.
HTTP is like sending a postcard with every message — you’ve got to fill out the address, stamp it, and send it off. WebSockets are like texting — quick, efficient, and straight to the point.
Setting Up Your First WebSocket Connection
Alright, enough with the theory — let’s get coding! We’ll start by setting up a basic WebSocket server and client to see how real-time communication works.
Step 1: Creating a WebSocket Server
We’ll use Node.js to create a simple WebSocket server. If you don’t have Node.js installed yet, go ahead and grab it from nodejs.org.
First, create a new directory for your project and initialize it:
mkdir websocket-demo
cd websocket-demo
npm init -y
Next, install the ws
package, which is a simple WebSocket implementation for Node.js:
npm install ws
Now, let’s create a WebSocket server in a file called server.js
:
const WebSocket = require("ws");
const wss = new WebSocket.Server({ port: 8080 });
wss.on("connection", (ws) => {
console.log("New client connected!");
ws.on("message", (message) => {
console.log(`Received message: ${message}`);
ws.send(`You said: ${message}`);
});
ws.on("close", () => {
console.log("Client disconnected");
});
});
console.log("WebSocket server is running on ws://localhost:8080");
Let’s break down what’s happening here:
wss.on('connection', ...)
: This event is triggered whenever a new client connects to the server. We’re logging a message to the console and setting up event listeners for incoming messages and when the client disconnects.ws.on('message', ...)
: This event is triggered whenever the server receives a message from a connected client. We’re logging the message and sending a response back to the client.ws.on('close', ...)
: This event is triggered when the client disconnects from the server.
Go ahead and run your server:
node server.js
You should see the message WebSocket server is running on ws://localhost:8080
in your terminal.
Step 2: Creating a WebSocket Client
Now that we have our server running, let’s create a client to connect to it. We’ll use plain HTML and JavaScript for this.
Create an index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>WebSocket Client</title>
</head>
<body>
<h1>WebSocket Client</h1>
<input id="messageInput" type="text" placeholder="Enter message" />
<button id="sendBtn">Send Message</button>
<pre id="output"></pre>
<script>
const ws = new WebSocket("ws://localhost:8080");
ws.onopen = () => {
document.getElementById("output").textContent = "Connected to server\n";
};
ws.onmessage = (event) => {
document.getElementById(
"output"
).textContent += `Server: ${event.data}\n`;
};
ws.onclose = () => {
document.getElementById("output").textContent +=
"Disconnected from server\n";
};
document.getElementById("sendBtn").onclick = () => {
const message = document.getElementById("messageInput").value;
ws.send(message);
document.getElementById("output").textContent += `You: ${message}\n`;
};
</script>
</body>
</html>
Here’s what’s happening in the client code:
const ws = new WebSocket('ws://localhost:8080');
: We’re creating a new WebSocket connection to our server.ws.onopen
: This event is triggered when the connection to the server is established. We update the output to show that we’re connected.ws.onmessage
: This event is triggered whenever we receive a message from the server. We display the message in the output.ws.onclose
: This event is triggered when the connection to the server is closed. We update the output to show that we’ve been disconnected.document.getElementById('sendBtn').onclick
: This event is triggered when the user clicks the “Send Message” button. We send the message typed in the input field to the server and display it in the output.
Now, open your index.html
file in a browser and give it a try. Type a message in the input field, hit “Send Message” and watch the real-time magic happen!
If you prefer to get finished code for this example, you can find it in this GitHub repository.
Advanced WebSocket Features
Now that you’ve got the basics down, let’s explore some advanced features you can implement with WebSockets to make your applications even more powerful.
Broadcasting Messages to All Clients
In many real-time applications, you’ll want to send messages to all connected clients, not just the one that sent the message. Let’s modify our server to broadcast messages:
wss.on("connection", (ws) => {
console.log("New client connected!");
ws.on("message", (message) => {
console.log(`Received message: ${message}`);
// Broadcast the message to all clients
wss.clients.forEach((client) => {
if (client.readyState === WebSocket.OPEN) {
client.send(`Broadcast: ${message}`);
}
});
});
ws.on("close", () => {
console.log("Client disconnected");
});
});
This will send the message to all connected clients, including the one that sent it.
Handling Binary Data
WebSockets aren’t just for text — they can also handle binary data, making them great for applications like file transfers or real-time video streaming. Let’s see how to send binary data:
Client-side:
const buffer = new ArrayBuffer(8);
const view = new DataView(buffer);
view.setUint8(0, 255); // Example: setting a byte
ws.send(buffer);
Server-side:
ws.on("message", (message) => {
if (message instanceof Buffer) {
console.log("Received binary data");
// Process binary data
} else {
console.log(`Received message: ${message}`);
}
});
Authentication
In a real-world application, you’ll likely need to authenticate users before allowing them to establish a WebSocket connection. Here’s a simple way to add authentication:
Client-side:
const token = "your-auth-token"; // This would be retrieved after logging in
const ws = new WebSocket(`ws://localhost:8080?token=${token}`);
Server-side:
wss.on("connection", (ws, req) => {
const token = new URLSearchParams(req.url.split("?")[1]).get("token");
if (token !== "your-auth-token") {
ws.close(4001, "Unauthorized");
return;
}
console.log("Authenticated client connected");
// Proceed with connection
});
This example demonstrates basic token-based authentication. In a production environment, you’d want to use a more secure method, such as JWT (JSON Web Tokens).
Common Use Cases for WebSockets
Now that you’re armed with WebSocket knowledge, let’s explore some common use cases where WebSockets shine.
Chat Applications
Real-time communication is the bread and butter of chat apps. WebSockets enable instant message delivery, typing indicators, and live updates — all without refreshing the page.
Live Feeds
Whether it’s news updates, sports scores, or social media feeds, WebSockets allow you to push updates to users in real-time, ensuring they’re always up to date.
Online Gaming
Multiplayer games rely on fast, continuous communication between players. WebSockets provide the low-latency connection needed for a smooth gaming experience.
Collaborative Tools
Tools like Google Docs or Trello use WebSockets to synchronize data in real-time across multiple users. This allows everyone to see changes as they happen, making collaboration seamless.
IoT (Internet of Things)
WebSockets are perfect for IoT applications where devices need to communicate with servers or each other in real-time. For example, you can monitor sensors and control devices instantaneously.
Conclusion
And there you have it — your comprehensive guide to WebSockets and real-time communication for modern web applications. Whether you’re building a chat app, a live feed, or the next big multiplayer game, WebSockets are the key to delivering a fast, responsive, and engaging user experience.
So, go forth and build with WebSockets! Your users will thank you for the real-time magic. And as always, here at Binary Brain, we’re cheering you on every step of the way — always with a smile and a dash of humor. Happy coding!