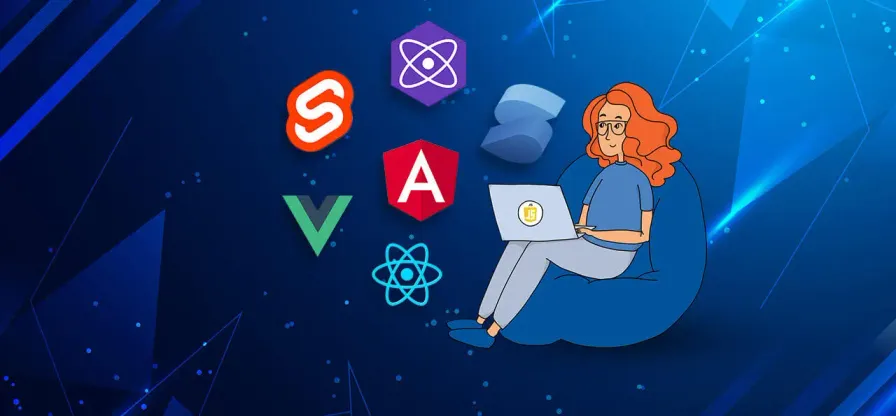
#webdev
Michał Uzdowski
An extensive guide to popular JavaScript frameworks
Welcome, fellow coders, to a (very) deep dive into the wild and wacky world of JavaScript frameworks! Clearly you have mastered all the JavaScript wisdom from the toms of The Wild World of JavaScript - One Line at a Time and now crave for more. Strap in, grab your snacks, and prepare to embark on a journey through the most popular frameworks that are shaping the future of web development. But beware: laughter-induced cramps are a common side effect of reading this article. Proceed with caution!
Frontend Frameworkds
Frontend frameworks are powerful tools that streamline the development of modern web applications by providing a structured approach to building user interfaces. These frameworks typically include libraries, tools, and conventions that facilitate tasks like routing, state management, and component composition, allowing developers to create dynamic and interactive experiences with ease. Popular frontend frameworks like React, Vue, and Angular offer a range of features and capabilities tailored to different development preferences and project requirements. Whether you’re building a single-page application, a progressive web app, or a complex enterprise application, frontend frameworks provide the building blocks and best practices needed to bring your ideas to life in the browser.
React
Ah, React – the diva of JavaScript frameworks, strutting onto the stage with all the confidence of a seasoned Broadway star. Let’s dive deeper into the mesmerizing world of React, where components reign supreme and the virtual DOM is the unsung hero of web development.
Overview
React is a JavaScript library developed by Facebook for building user interfaces. It follows a component-based architecture, where UIs are divided into reusable and independent components, each responsible for rendering a specific part of the UI.
Concepts
- Components: The building blocks of React applications, components encapsulate both the UI and behavior of a particular part of the application.
- Virtual DOM: React creates a virtual representation of the DOM in memory, which it uses to efficiently update the actual DOM when state changes occur.
- JSX: A syntax extension for JavaScript, JSX allows you to write HTML-like code within JavaScript, making it easier to create React components.
Conventions
- Single Source of Truth: React encourages a single source of truth for managing state, typically stored in a parent component and passed down to child components via props.
- Unidirectional Data Flow: Data flows in a single direction in React applications, from parent to child components. This ensures predictable and maintainable code.
Pros
- Component Reusability: React’s component-based architecture promotes code reusability, making it easier to maintain and refactor code.
- Virtual DOM: React’s virtual DOM allows for efficient updates to the actual DOM, resulting in improved performance and a smoother user experience.
- Large Ecosystem: React has a vast ecosystem of libraries, tools, and community support, making it easy to find solutions to common problems.
Cons
- Learning Curve: React has a steep learning curve, especially for developers new to concepts like JSX, virtual DOM, and state management.
- JSX Syntax: Some developers find JSX syntax unfamiliar and may face challenges integrating it into existing projects or workflows.
- Boilerplate Code: React applications often require boilerplate code for setting up components, state management, and routing, which can increase development time.
React Example
// HelloComponent.jsx
import React from "react";
const HelloComponent = ({ name }) => {
return <div>Hello, {name}!</div>;
};
export default HelloComponent;
With its component-based architecture, virtual DOM magic, and vibrant community, React continues to dazzle developers and delight users around the globe. So go forth, create, and let your imagination run wild with React!
React DocumentationNext.js
Welcome to the realm of Next.js – the JavaScript framework that’s taking server-side rendering to new heights and blazing trails in the world of modern web development. Prepare to embark on an exciting journey through the features, concepts, and capabilities that make Next.js a top choice for building dynamic and performant web applications.
Overview
Next.js is a powerful JavaScript framework for building server-side rendered and statically generated web applications. Developed by Vercel, Next.js offers a comprehensive platform for building fast, scalable, and SEO-friendly web experiences, with features like automatic code splitting, hot module replacement, and built-in routing.
Concepts
- Server-Side Rendering (SSR): Next.js supports server-side rendering out of the box, allowing you to pre-render pages on the server and deliver fully rendered HTML to the client. This results in faster initial page loads and improved SEO.
- Static Site Generation (SSG): In addition to SSR, Next.js offers static site generation, where pages are pre-built at build time and served as static HTML files. This approach enables lightning-fast page loads and efficient content delivery.
- File-Based Routing: Next.js uses a file-based routing system, where each page of your application corresponds to a file in the
app
(previouslypages
) directory. This makes it easy to create and organize routes without the need for complex configuration. - API Routes: Next.js provides built-in support for creating API routes, allowing you to define serverless functions that handle HTTP requests and interact with your application’s data and services.
Conventions
- Pages Directory: Next.js uses the
app
directory as the entry point for defining routes in your application. Each folder or JavaScript, TypeScript, or Markdown file in theapp
directory represents a route in your application. - Static Files: Next.js allows you to serve static files like images, CSS, and JavaScript assets using the
public
directory. Files in thepublic
directory are served under the root URL of your application. - Dynamic Routes: A Dynamic Segment can be created by wrapping a folder’s name in square brackets:
[folderName]
. For example,[id]
or[slug]
and then passed as theparams
prop to layout, page, route, and generateMetadata functions.
Pros
- Performance: Next.js offers best-in-class performance, with features like automatic code splitting, optimized image loading, and efficient server-side rendering.
- Developer Experience: Next.js provides a delightful developer experience, with features like hot module replacement, fast refresh, and built-in TypeScript support.
- SEO-Friendly: Next.js is SEO-friendly out of the box, with support for server-side rendering and static site generation, ensuring that your content is easily discoverable by search engines.
Cons
- Complexity: Next.js can be complex for beginners, especially those new to server-side rendering and static site generation concepts.
- Learning Curve: While Next.js provides extensive documentation and tutorials, developers may need some time to familiarize themselves with its conventions and APIs.
- Server-Side Requirements: Next.js applications require a Node.js server to run, which may not be suitable for all hosting environments.
Next.js Example
// app/page.js
import React from "react";
const HomePage = () => {
return (
<div>
<h1>Welcome to Next.js!</h1>
<p>Build fast and scalable web applications with Next.js.</p>
</div>
);
};
export default HomePage;
So there you have it – a thrilling exploration of Next.js, the JavaScript framework that’s redefining the future of web development. With its powerful features, intuitive conventions, and top-notch performance, Next.js is leading the charge towards a faster, more scalable web. So fire up your engines, strap in, and get ready to take your web applications to the next level with Next.js!
Next.js DocumentationVue
Let’s step into the charming world of Vue.js – the JavaScript framework that’s as refreshing as a summer breeze and as versatile as a Swiss army knife. Here’s everything you need to know about Vue.js, from its elegant syntax to its intuitive reactivity system.
Overview
Vue.js is a progressive JavaScript framework for building user interfaces. It’s designed to be incrementally adoptable, meaning you can start small with individual components and gradually scale up to full-blown single-page applications.
Concepts
- Components: Like React, Vue.js revolves around components – self-contained units of UI that can be reused and composed to build complex interfaces.
- Reactivity: Vue.js leverages a reactive data-binding system, which automatically updates the DOM when data changes, eliminating the need for manual DOM manipulation.
- Directives: Vue.js includes a set of built-in directives that allow you to add dynamic behavior to HTML elements. For example, the
v-if
directive conditionally renders elements based on a boolean value.
Conventions
- Vue Instance: Every Vue.js application starts with a Vue instance, which serves as the root of the application and provides access to the Vue API.
- Single File Components: Vue.js allows you to encapsulate a component’s template, JavaScript, and styles in a single
.vue
file, making it easier to organize and maintain code. - Computed Properties and Watchers: Vue.js provides features like computed properties and watchers for reacting to changes in data and performing side effects.
Pros
- Gentle Learning Curve: Vue.js has a gentle learning curve, making it easy for beginners to get started and for seasoned developers to pick up quickly.
- Versatility: Vue.js is versatile and can be used to build anything from simple UI components to full-fledged single-page applications.
- Size: Vue.js has a small file size and minimal overhead, making it ideal for projects where performance is a priority.
Cons
- Ecosystem Size: While Vue.js has a growing ecosystem and community, it may not be as extensive as frameworks like React or Angular.
- Maturity: Vue.js is still relatively young compared to other frameworks, which means it may not have as many established best practices or libraries.
Vue.js Example
<!-- HelloComponent.vue -->
<template>
<div>Hello, {{ name }}!</div>
</template>
<script>
export default {
props: ["name"],
};
</script>
<style scoped>
/* Scoped styles for HelloComponent */
div {
color: green;
}
</style>
There, there - a delightful introduction to Vue.js, the JavaScript framework that’s as charming as it is powerful. With its simplicity, versatility, and focus on developer experience, Vue.js continues to win the hearts of developers worldwide. So go ahead, give Vue.js a try, and let your creativity soar!
Vue DocumentationAngular
It’s time to face the robust world of Angular – the superhero of JavaScript frameworks, ready to save the day with its powerful features and comprehensive tooling. Get ready to explore the ins and outs of Angular, from its opinionated architecture to its built-in solutions for building large-scale applications.
Overview
Angular is a TypeScript-based JavaScript framework for building client-side web applications. Developed and maintained by Google, Angular provides a full-featured platform for developing web applications, including tools for routing, forms, HTTP requests, and more.
Concepts
- Modules: Angular applications are organized into modules, which encapsulate related components, directives, pipes, and services. Modules help keep code organized and modular, allowing for easy reuse and maintainability.
- Components: Components are the building blocks of Angular applications, representing elements of the UI. Each component consists of a template, which defines the component’s view, along with a class, which contains the component’s logic.
- Dependency Injection: Angular’s dependency injection system allows components and services to declare their dependencies and have them injected by the Angular injector. This promotes loose coupling and makes testing easier.
Conventions
- TypeScript: Angular applications are typically written in TypeScript, a superset of JavaScript that adds static typing and other advanced features to the language.
- Angular CLI: The Angular CLI (Command Line Interface) is a powerful tool for scaffolding, building, and managing Angular applications. It provides commands for generating components, services, modules, and more, streamlining the development process.
- RxJS: Angular uses RxJS, a reactive programming library, for handling asynchronous operations and managing streams of data.
Pros
- Full-Featured: Angular provides a comprehensive platform for building web applications, including built-in solutions for routing, forms, HTTP requests, and more.
- Strong Typing: TypeScript’s static typing adds a layer of safety and predictability to Angular applications, helping catch errors at compile-time rather than runtime.
- Official Support: Angular is developed and maintained by Google, ensuring regular updates, long-term support, and extensive documentation.
Cons
- Steep Learning Curve: Angular has a steep learning curve, especially for developers new to TypeScript and reactive programming concepts.
- Boilerplate Code: Angular applications often require a significant amount of boilerplate code, which can make getting started more challenging compared to other frameworks.
- Bundle Size: Angular applications tend to have larger bundle sizes compared to other frameworks, which can impact performance, especially on mobile devices.
Angular Example
// hello.component.ts
import { Component } from "@angular/core";
@Component({
selector: "app-hello",
template: "<div>Hello, {{ name }}!</div>",
})
export class HelloComponent {
name = "Angular";
}
And here is a deep dive into the powerful world of Angular, where building robust web applications is as easy as assembling a team of superheroes. With its comprehensive features, strong typing, and official support from Google, Angular continues to be a top choice for developers tackling ambitious projects. So don your cape, embrace the power of Angular, and let your web applications soar to new heights!
Angular DocumentationAstro
Let’s embark on a thrilling journey into the cosmos of Astro – the futuristic framework that’s rewriting the rules of web development. Get ready to explore the cutting-edge features and innovative concepts that make Astro a game-changer in the world of static site generation and beyond.
Overview
Astro is a modern framework for building fast, scalable websites and web applications. It combines the best features of static site generation and server-side rendering, allowing developers to create dynamic, interactive experiences that load instantly and perform flawlessly.
Concepts
- Static Site Generation: Astro generates static HTML, CSS, and JavaScript files at build time, resulting in fast page loads and improved performance.
- Partial Hydration: Astro hydrates only the interactive parts of the page on the client side, while the rest of the content remains static. This allows for faster initial page loads and smoother transitions between pages.
- Framework Agnostic: Astro is framework agnostic, meaning you can use your favorite front-end framework, such as React, Vue.js, or Svelte, to build your Astro applications.
- Optimized Builds: Astro optimizes your application’s assets and resources for production, resulting in smaller bundle sizes and faster load times.
Conventions
- Component-Based Architecture: Astro follows a component-based architecture, where UI elements are encapsulated into reusable components. This promotes code reusability and maintainability.
- Single File Components: Similar to Vue.js, Astro allows you to define components using single file
.astro
files, which contain HTML, CSS, and JavaScript code. - Markdown Support: Astro has built-in support for Markdown files, allowing you to easily create and manage content for your website or application.
Pros
- Blazing Fast Performance: Astro generates static files at build time, resulting in lightning-fast page loads and improved performance.
- Partial Hydration: Astro’s partial hydration feature ensures that only the interactive parts of the page are hydrated on the client side, reducing initial page load times and improving overall user experience.
- Framework Agnostic: Astro is compatible with popular front-end frameworks like React, Vue.js, and Svelte, giving developers the flexibility to choose the tools that best suit their needs.
Cons
- Learning Curve: While Astro’s concepts are relatively straightforward, developers may need some time to familiarize themselves with its unique approach to static site generation and hydration.
- Limited Ecosystem: As a relatively new framework, Astro’s ecosystem and community support may not be as extensive as more established frameworks like React or Vue.js.
- Complexity: Building complex applications with Astro may require additional configuration and setup, especially when integrating with external APIs or services.
Astro Example
<!-- HelloComponent.astro -->
---
import React from 'react';
import { renderToString } from 'react-dom/server';
import App from './App.astro';
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Hello, Astro!</title>
</head>
<body>
<div id="app">{renderToString(<App />)}</div>
</body>
</html>
So here we land after a thrilling exploration of Astro, the framework that’s boldly going where no framework has gone before. With its innovative approach to static site generation and dynamic hydration, Astro is poised to revolutionize the way we build websites and web applications. So strap in, hold on tight, and get ready to launch your projects into orbit with Astro!
Astro DocumentationGatsby
Welcome to the captivating universe of Gatsby – the static site generator that’s redefining the way we build modern web experiences. Prepare to embark on an exciting journey through the features, concepts, and magic that make Gatsby a powerhouse in the world of static site generation and beyond.
Overview
Gatsby is a blazing-fast static site generator for React, designed to help developers build incredibly fast websites and web applications. With its robust ecosystem of plugins and a powerful data layer, Gatsby empowers developers to create dynamic, content-rich websites that load instantly and rank higher in search engine results.
Concepts
- Static Site Generation (SSG): Gatsby leverages the power of static site generation to pre-render pages at build time, resulting in HTML, CSS, and JavaScript files that can be served directly to the client. This approach eliminates the need for server-side processing and delivers lightning-fast performance.
- GraphQL Data Layer: Gatsby’s data layer is powered by GraphQL, a powerful query language for fetching and manipulating data. With Gatsby’s GraphQL API, developers can query data from multiple sources, including local files, databases, REST APIs, and CMS platforms, and seamlessly integrate it into their components.
- Plugin Ecosystem: Gatsby boasts a vast ecosystem of plugins that extend its functionality and streamline common tasks like image optimization, SEO management, and content management. With over a thousand plugins available, developers can customize and enhance their Gatsby projects to suit their specific needs.
- React-Based Architecture: Gatsby is built on top of React, the popular JavaScript library for building user interfaces. This allows developers to leverage the power of React’s component-based architecture and declarative programming model to create interactive and dynamic web experiences.
Conventions
- File-Based Routing: Gatsby uses a file-based routing system, where each page of your website corresponds to a file in the
pages
directory. This makes it easy to create and organize routes without the need for complex configuration. - Node.js Ecosystem: Gatsby is built on top of Node.js, the JavaScript runtime environment. This allows developers to leverage the rich ecosystem of Node.js libraries and tools to extend and customize their Gatsby projects.
- Optimized Build Process: Gatsby’s build process is highly optimized, with features like automatic code splitting, image optimization, and lazy loading built-in. This ensures that Gatsby sites are fast, efficient, and optimized for performance.
Pros
- Performance: Gatsby generates static assets that are optimized for speed and performance, resulting in blazing-fast page loads and superior user experiences.
- Developer Experience: Gatsby provides a delightful developer experience, with features like hot module replacement, fast refresh, and built-in TypeScript support. The intuitive CLI and documentation make it easy to get started with Gatsby and build complex websites with ease.
- SEO-Friendly: Gatsby sites are SEO-friendly out of the box, with features like server-side rendering and automatic generation of sitemaps and meta tags. This ensures that your content is easily discoverable by search engines and ranks higher in search results.
Cons
- Learning Curve: While Gatsby’s concepts are relatively straightforward, developers may need some time to familiarize themselves with its unique architecture and conventions, especially if they are new to static site generation or GraphQL.
- Plugin Compatibility: While Gatsby’s plugin ecosystem is vast and diverse, not all plugins are created equal. Developers may encounter compatibility issues or conflicts between plugins, requiring careful consideration and testing.
- Build Times: Gatsby’s build process can be time-consuming, especially for large projects with extensive data sources or complex build configurations. Optimizing build times and reducing build-related bottlenecks can require careful optimization and tuning.
Gatsby Example
// pages/index.js
import React from "react";
import { graphql } from "gatsby";
const HomePage = ({ data }) => {
const { title, description } = data.site.siteMetadata;
return (
<div>
<h1>{title}</h1>
<p>{description}</p>
</div>
);
};
export const query = graphql`
query {
site {
siteMetadata {
title
description
}
}
}
`;
export default HomePage;
So… - Gatsby, the static site generator that’s transforming the web development landscape. With its unparalleled performance, rich ecosystem, and delightful developer experience, Gatsby continues to captivate developers and push the boundaries of what’s possible on the web. So dive in, unleash the magic of Gatsby, and create extraordinary web experiences that amaze and inspire!
Gatsby DocumentationSolid
Hold on to your hats as we delve into the world of Solid – the JavaScript library that’s as sturdy as a rock and as flexible as a gymnast. Get ready to explore the robust features and unique concepts that make Solid a formidable contender in the realm of reactive UI libraries.
Overview
Solid is a declarative JavaScript library for building fast and reactive user interfaces. Inspired by React and other reactive programming paradigms, Solid offers a lightweight yet powerful solution for creating dynamic web applications with minimal overhead.
Concepts
- Reactivity: Solid embraces a reactive programming model, where UI components automatically update in response to changes in data. This reactive system eliminates the need for manual DOM manipulation and ensures that your UI stays in sync with your application’s state.
- Fine-Grained Reactive Updates: Unlike other frameworks that re-render entire components on state changes, Solid performs fine-grained updates at the DOM level, resulting in faster performance and smoother user experiences.
- Component Composition: Solid encourages component-based architecture, allowing you to break your UI into reusable and composable components that can be easily shared and maintained.
- Efficient Rendering: Solid employs a virtual DOM diffing algorithm to efficiently update the DOM only when necessary, minimizing unnecessary re-renders and optimizing performance.
Conventions
- JSX-Like Syntax: Similar to React, Solid uses JSX-like syntax for defining components, making it easy to create and compose UI elements.
- Reactive State Management: Solid provides built-in utilities for managing reactive state, allowing you to define reactive variables, computed values, and reactive effects to handle side effects.
- Event Handling: Solid offers a streamlined approach to event handling, with built-in directives for handling DOM events and updating state in response.
Pros
- Performance: Solid’s fine-grained reactivity and efficient rendering make it one of the fastest reactive UI libraries available, delivering blazing-fast performance even in complex applications.
- Lightweight: Solid has a small footprint and minimal overhead, making it ideal for projects where performance and bundle size are critical factors.
- Predictable Behavior: Solid’s declarative API and reactive programming model result in predictable and maintainable code, making it easier to reason about and debug your applications.
Cons
- Learning Curve: While Solid’s concepts are relatively straightforward, developers may need some time to familiarize themselves with its reactive programming model and JSX-like syntax.
- Ecosystem Size: As a newer library, Solid’s ecosystem and community support may not be as extensive as more established frameworks like React or Vue.js.
- Compatibility: Solid’s approach to reactivity and rendering may differ from other libraries, which could lead to compatibility issues when integrating with third-party libraries or frameworks.
Solid Example
// HelloComponent.jsx
import { createSignal } from "solid-js";
const HelloComponent = () => {
const [name, setName] = createSignal("Solid");
return (
<div>
<h1>Hello, {name()}!</h1>
<input
type="text"
value={name()}
onInput={(e) => setName(e.target.value)}
/>
</div>
);
};
export default HelloComponent;
Solid, the reactive UI library that’s redefining the way we build user interfaces. With its fine-grained reactivity, efficient rendering, and lightweight footprint, Solid is poised to revolutionize the world of front-end development. So strap in, buckle up, and get ready to rock with Solid!
Solid.js DocumentationSvelte
Welcome to Svelte – the magical JavaScript framework that’s reshaping the landscape of front-end development with its innovative approach to building reactive user interfaces. Join us as we embark on an enchanting journey through the features, concepts, and wonders that make Svelte a true gem in the realm of web development.
Overview
Svelte is a radical new framework for building web applications. Unlike traditional frameworks that run in the browser, Svelte shifts much of the work to the build step, resulting in smaller, faster, and more efficient code. With its unique compiler approach, Svelte transforms declarative components into highly optimized JavaScript at build time, delivering lightning-fast performance and a smaller bundle size.
Concepts
- Reactivity: Svelte embraces a reactive programming model, where components automatically update in response to changes in data. Unlike other frameworks that rely on virtual DOM diffing, Svelte compiles reactive updates directly into imperative code, resulting in faster rendering and smoother animations.
- Component-Based Architecture: Like other modern frameworks, Svelte promotes a component-based architecture, allowing you to encapsulate UI elements into reusable and composable components. However, Svelte takes it a step further by eliminating the need for a virtual DOM, resulting in simpler and more efficient components.
- No Virtual DOM: One of the key innovations of Svelte is its lack of a virtual DOM. Instead of diffing virtual DOM trees, Svelte generates code that directly manipulates the DOM, resulting in faster rendering and reduced memory overhead.
- Build-time Compilation: Svelte’s compiler analyzes and optimizes your code at build time, transforming declarative components into highly efficient JavaScript code. This approach eliminates the need for runtime overhead and enables Svelte applications to achieve unparalleled performance.
Conventions
- Single File Components: Svelte components are typically defined in single file
.svelte
format, which encapsulates HTML, CSS, and JavaScript code in a single file. This makes it easy to create and manage components without the need for separate files. - Reactive Statements: Svelte provides built-in directives and reactive statements for handling reactivity, including
{#if}
blocks,{#each}
blocks, and{#await}
blocks. These reactive statements allow you to conditionally render content, iterate over arrays, and await asynchronous operations with ease. - Event Handling: Svelte offers a streamlined approach to event handling, with built-in event modifiers and event forwarding. This makes it easy to handle user interactions and update component state without boilerplate code.
Pros
- Performance: Svelte delivers exceptional performance by eliminating the virtual DOM and optimizing code at build time. This results in faster rendering, smaller bundle sizes, and reduced memory overhead.
- Developer Experience: Svelte provides a delightful developer experience, with features like automatic code splitting, hot module replacement, and built-in TypeScript support. The intuitive syntax and straightforward conventions make it easy to get started with Svelte and build complex applications with ease.
- Size: Svelte applications have smaller bundle sizes compared to other frameworks, thanks to its efficient compiler and lack of runtime overhead. This makes Svelte ideal for projects where performance and size are critical factors.
Cons
- Learning Curve: While Svelte’s concepts are relatively straightforward, developers may need some time to adjust to its unique approach to reactive programming and build-time compilation.
- Ecosystem Size: As a newer framework, Svelte’s ecosystem and community support may not be as extensive as more established frameworks like React or Vue.js. However, the community is growing rapidly, with an increasing number of libraries and tools being developed for Svelte.
- Tooling: Svelte’s tooling ecosystem is still evolving, with fewer options compared to other frameworks. However, the official Svelte compiler and dev tools provide everything you need to build and deploy Svelte applications with confidence.
Svelte Example
<!-- Hello.svelte -->
<script>
let name = "Svelte";
</script>
<h1>Hello, {name}!</h1>
<style>
h1 {
color: blue;
}
</style>
That wraps up a captivating exploration of Svelte, the JavaScript framework that’s rewriting the rules of front-end development. With its innovative approach to reactive programming, build-time compilation, and streamlined syntax, Svelte is leading the charge towards a faster, more efficient web. So dive in, embrace the magic of Svelte, and unlock the full potential of your web applications!
Svelte DocumentationBackend Frameworks
Backend JavaScript frameworks are powerful tools for building the server-side logic and infrastructure of web applications using JavaScript. These frameworks provide developers with a comprehensive set of features and conventions for handling tasks such as routing, database interactions, authentication, and API development. By leveraging the capabilities of JavaScript runtime environments like Node.js, backend JavaScript frameworks like Express.js, Nest.js, and Fastify enable developers to build scalable and efficient backend systems that power modern web applications. With features such as middleware support, dependency injection, and robust error handling, backend JavaScript frameworks streamline the development process and promote code maintainability and scalability. Whether you’re building a RESTful API, a microservices architecture, or a full-stack web application, backend JavaScript frameworks offer the tools and flexibility needed to build robust and reliable backend systems.
Express.js
Meet Express.js – the powerhouse of server-side web development in the JavaScript ecosystem. Get ready to explore the robust features, flexible architecture, and unparalleled performance that make Express.js the go-to choice for building fast, scalable, and reliable web applications.
Overview
Express.js is a minimalist web application framework for Node.js, designed to make building web servers and APIs fast, simple, and expressive. With its lightweight and unopinionated approach, Express.js provides developers with the flexibility and freedom to design and structure their applications according to their specific needs.
Concepts
- Middleware: Express.js is built around the concept of middleware, which are functions that have access to the request and response objects. Middleware functions can perform tasks such as parsing request bodies, authenticating users, and handling errors.
- Routing: Express.js provides a powerful routing API that allows you to define routes for handling HTTP requests. Routes can be defined for different HTTP methods (GET, POST, PUT, DELETE) and URL patterns, making it easy to create RESTful APIs and web applications.
- Template Engines: While Express.js itself does not include a built-in template engine, it provides support for integrating with popular template engines like Pug, EJS, and Handlebars. Template engines allow you to dynamically generate HTML content based on data from your application.
- Error Handling: Express.js provides robust error handling mechanisms, including built-in middleware for handling errors and a global error handler that catches unhandled errors and sends appropriate responses to clients.
Conventions
- Routing: Express.js follows a convention-over-configuration approach to routing, allowing you to define routes using simple and intuitive syntax. Routes can be defined using methods like
app.get()
,app.post()
,app.put()
, andapp.delete()
. - Middleware Pipeline: Express.js middleware functions are executed in a sequential order, with each middleware function having the ability to modify the request and response objects or pass control to the next middleware in the pipeline.
- Error Handling: Express.js provides built-in middleware for handling common types of errors, such as 404 Not Found errors and 500 Internal Server errors. Additionally, you can define custom error-handling middleware to handle application-specific errors.
Pros
- Simplicity: Express.js has a simple and intuitive API, making it easy to get started with building web servers and APIs.
- Flexibility: Express.js is unopinionated and flexible, allowing you to choose the tools and libraries that best suit your project requirements.
- Performance: Express.js is known for its excellent performance, with low overhead and fast response times even under heavy loads.
Cons
- Boilerplate Code: While Express.js provides a lightweight foundation for building web servers and APIs, developers may need to write additional code to handle common tasks like authentication, input validation, and database access.
- Lack of Opinionation: Express.js leaves many architectural decisions up to the developer, which can lead to inconsistency and lack of standardization across projects.
- Middleware Ordering: Managing the order and execution of middleware functions can be challenging, especially in larger applications with complex middleware pipelines.
Express.js Example
const express = require("express");
const app = express();
app.get("/", (req, res) => {
res.send("Hello, Express!");
});
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
So there it is – an exhilarating journey through the world of Express.js, the backbone of server-side web development in JavaScript. With its simplicity, flexibility, and unmatched performance, Express.js continues to be the preferred choice for developers building web servers and APIs. So fire up your Node.js environment, unleash the power of Express.js, and take your web applications to new heights!
Express DocumentationFastify
Welcome to Fastify – the lightning-fast web framework for Node.js that’s revolutionizing the way we build high-performance APIs and web applications. Join us on a thrilling journey through the features, concepts, and optimizations that make Fastify a top choice for developers seeking speed, efficiency, and scalability.
Overview
Fastify is a web framework for Node.js focused on speed and low overhead. Built with performance in mind, Fastify offers a robust foundation for building fast and efficient web servers and APIs. With its streamlined architecture and minimalist approach, Fastify enables developers to create blazing-fast applications that scale effortlessly.
Concepts
- Asynchronous Architecture: Fastify is built around an asynchronous, event-driven architecture, allowing it to handle high loads and concurrent requests with ease. By leveraging asynchronous programming techniques and non-blocking I/O, Fastify maximizes throughput and minimizes latency.
- Schema-Based Validation: Fastify provides built-in support for schema-based request and response validation, allowing you to define the shape and structure of your data using JSON Schema. This helps ensure that incoming requests are valid and properly formatted before being processed by your application.
- Plugin System: Fastify features a powerful plugin system that allows you to extend and customize its functionality with ease. With over a hundred official plugins available, as well as a vibrant ecosystem of community-contributed plugins, Fastify offers unparalleled flexibility and extensibility.
- Serialization and Deserialization: Fastify provides built-in support for serialization and deserialization of data, allowing you to easily convert between JavaScript objects and JSON, XML, or other formats. This makes it easy to work with different data formats and integrate with external APIs and services.
Conventions
- Route Handlers: Fastify uses route handlers to define the behavior of your web server or API endpoints. Route handlers are asynchronous functions that receive the request and response objects as arguments and can perform any necessary processing before sending a response back to the client.
- Middleware: Fastify supports middleware functions that can be used to perform common tasks such as logging, authentication, and error handling. Middleware functions are executed in the order they are registered and can modify the request and response objects as needed.
- Error Handling: Fastify provides robust error handling mechanisms, including built-in support for throwing and catching errors within route handlers and middleware functions. Additionally, Fastify allows you to define custom error handlers to handle application-specific errors and return appropriate responses to clients.
Pros
- Performance: Fastify is renowned for its exceptional performance, with benchmarks consistently ranking it among the fastest web frameworks for Node.js. By minimizing overhead and optimizing critical path code, Fastify delivers unparalleled speed and efficiency.
- Scalability: Fastify’s lightweight and efficient architecture makes it ideal for building scalable web applications that can handle high loads and concurrent requests with ease. With built-in support for clustering and horizontal scaling, Fastify empowers developers to build applications that can grow with their needs.
- Flexibility: Fastify offers a high degree of flexibility and customization, allowing you to tailor your application to your specific requirements. Whether you’re building a simple API or a complex web application, Fastify provides the tools and features you need to get the job done.
Cons
- Learning Curve: While Fastify’s concepts are relatively straightforward, developers may need some time to familiarize themselves with its asynchronous programming model and plugin system. Additionally, Fastify’s minimalist approach may require developers to write more code to implement certain features compared to more opinionated frameworks.
- Community and Ecosystem: While Fastify has a growing community of users and contributors, it may not have the same level of community support or ecosystem as more established frameworks like Express.js. Developers may need to rely more on official documentation and resources when working with Fastify.
- Middleware Ordering: Managing the order and execution of middleware functions can be challenging, especially in larger applications with complex middleware pipelines. Developers may need to carefully orchestrate middleware to ensure proper execution and error handling.
Fastify Example
const fastify = require("fastify")();
fastify.get("/", async (request, reply) => {
return { hello: "world" };
});
fastify.listen(3000, (err) => {
if (err) throw err;
console.log(`Server is running on http://localhost:3000`);
});
Now you know about Fastify, the lightning-fast web framework that’s raising the bar for Node.js development. With its exceptional performance, flexible architecture, and robust features, Fastify continues to impress developers and power some of the fastest web applications on the planet. So strap in, rev up your engines, and experience the thrill of building high-performance web applications with Fastify!
Fastify DocumentationNest.js
Nest.js – the progressive Node.js framework that’s redefining server-side application development with TypeScript. Prepare to embark on an enlightening journey through the features, concepts, and innovations that make Nest.js a top choice for developers seeking scalability, maintainability, and productivity.
Overview
Nest.js is a progressive Node.js framework for building efficient, reliable, and scalable server-side applications. Built with TypeScript and inspired by Angular, Nest.js leverages modern JavaScript features and design patterns to provide a robust foundation for building enterprise-grade applications. Whether you’re building RESTful APIs, GraphQL servers, or full-stack web applications, Nest.js offers a comprehensive solution for all your server-side needs.
Concepts
- Modular Architecture: Nest.js encourages a modular architecture, where applications are organized into modules that encapsulate related functionality. Each module can contain controllers, services, providers, and other components, making it easy to manage complexity and scale your application as it grows.
- Dependency Injection: Nest.js utilizes the dependency injection pattern to manage the creation and resolution of dependencies within your application. This promotes loose coupling, testability, and code reusability, allowing you to easily swap out implementations or mock dependencies for testing.
- Middleware: Nest.js provides built-in support for middleware, which are functions that have access to the request and response objects and can perform tasks like logging, authentication, and error handling. Middleware functions can be applied globally, at the module level, or to individual routes, providing fine-grained control over request processing.
- Decorators and Metadata: Nest.js leverages decorators and metadata to define and configure components such as controllers, providers, and routes. Decorators are a powerful tool for adding metadata to classes, methods, and properties, enabling Nest.js to automatically discover and wire up components at runtime.
Conventions
- Directory Structure: Nest.js follows a convention-over-configuration approach to directory structure, where files and folders are organized according to their role and purpose within the application. This makes it easy to navigate and understand the structure of a Nest.js project, even as it grows in complexity.
- Decorators and Annotations: Nest.js uses decorators extensively to annotate classes, methods, and properties with metadata that defines their behavior and configuration. By following established naming conventions and patterns, developers can quickly identify and understand the purpose of different components within a Nest.js application.
- Module Composition: Nest.js encourages the composition of modules, where smaller modules are combined to create larger, more complex applications. This promotes code reuse, separation of concerns, and maintainability, allowing developers to build scalable and maintainable applications with ease.
Pros
- TypeScript Support: Nest.js is built with TypeScript, a statically typed superset of JavaScript that provides powerful tooling, type checking, and code navigation features. This enables developers to catch errors early, write more reliable code, and enhance productivity.
- Modularity: Nest.js promotes a modular architecture, where applications are organized into cohesive modules that encapsulate related functionality. This makes it easy to manage complexity, scale your application, and reuse code across different parts of your project.
- Scalability: Nest.js is designed for scalability, with built-in support for features like dependency injection, middleware, and asynchronous programming. This allows you to build high-performance, scalable applications that can handle large numbers of concurrent requests with ease.
Cons
- Learning Curve: While Nest.js’s concepts are relatively straightforward, developers may need some time to familiarize themselves with its conventions, patterns, and best practices, especially if they are new to TypeScript or server-side development.
- Opinionated Approach: Nest.js follows a opinionated approach to application architecture and design, which may not be suitable for all projects or development styles. Developers may need to adapt their coding practices and workflows to align with Nest.js’s conventions.
- Performance Overhead: While Nest.js offers many features and benefits, some developers may find that its emphasis on modularity and abstraction comes with a performance overhead compared to more lightweight frameworks or libraries.
Nest.js Example
// src/app.controller.ts
import { Controller, Get } from "@nestjs/common";
@Controller()
export class AppController {
@Get()
getHello(): string {
return "Hello, Nest.js!";
}
}
And that’s Nest.js, the progressive Node.js framework that’s setting new standards for server-side application development. With its modern features, expressive syntax, and unparalleled productivity, Nest.js continues to empower developers and delight users around the world. So dive in, embrace the power of Nest.js, and elevate your server-side development journey to new heights!
Nest.js DocumentationNuxt.js
Welcome Nuxt.js – the Vue.js framework that makes building server-side rendered (SSR) and static web applications a breeze. Join us on an exciting journey through the features, concepts, and magic that make Nuxt.js a favorite among developers seeking simplicity, speed, and scalability.
Overview
Nuxt.js is a progressive Vue.js framework for building modern web applications. With its opinionated yet flexible approach, Nuxt.js streamlines the development process by providing built-in solutions for common tasks like routing, state management, and server-side rendering. Whether you’re building a dynamic single-page application (SPA), a server-rendered application (SSR), or a static site, Nuxt.js has you covered.
Concepts
- Vue.js Integration: Nuxt.js builds upon Vue.js, the popular JavaScript framework for building user interfaces. By leveraging Vue.js’s component-based architecture and reactive data binding, Nuxt.js enables developers to create interactive and dynamic web applications with ease.
- Server-Side Rendering (SSR): One of Nuxt.js’s key features is its support for server-side rendering, which allows you to pre-render Vue.js components on the server and send fully-rendered HTML to the client. This improves performance, enables better SEO, and ensures a consistent user experience across different devices and environments.
- Routing and Layouts: Nuxt.js provides a powerful routing system that automatically generates routes based on your file structure. Additionally, Nuxt.js introduces the concept of layouts, which allow you to define common page layouts and apply them to multiple pages in your application.
- Static Site Generation (SSG): In addition to server-side rendering, Nuxt.js supports static site generation, where pages are pre-rendered at build time and served as static HTML files. This approach is ideal for content-focused websites, blogs, and documentation sites that don’t require dynamic server-side rendering.
Conventions
- File-Based Routing: Nuxt.js follows a convention-over-configuration approach to routing, where each Vue.js component in the
pages
directory corresponds to a route in your application. This makes it easy to organize and structure your routes without the need for explicit configuration. - Vue.js Lifecycle Hooks: Nuxt.js provides built-in support for Vue.js lifecycle hooks, allowing you to hook into different stages of a component’s lifecycle and perform tasks like data fetching, server-side rendering, and client-side initialization.
- Vuex Integration: Nuxt.js seamlessly integrates with Vuex, the official state management library for Vue.js. This allows you to manage application state in a centralized store and access it from any component in your application.
Pros
- Simplicity: Nuxt.js simplifies the development process by providing built-in solutions for common tasks like routing, state management, and server-side rendering. This reduces boilerplate code and allows developers to focus on building features rather than managing infrastructure.
- Performance: Nuxt.js improves performance by pre-rendering pages on the server and serving static HTML files to the client. This results in faster page loads, better SEO, and a smoother user experience.
- Versatility: Nuxt.js supports a wide range of deployment options, including server-side rendering, static site generation, and single-page applications. This makes it suitable for a variety of use cases, from simple websites to complex web applications.
Cons
- Learning Curve: While Nuxt.js’s concepts are relatively straightforward, developers may need some time to familiarize themselves with its conventions and best practices, especially if they are new to server-side rendering or static site generation.
- Flexibility vs. Opinions: Nuxt.js’s opinionated approach may not be suitable for all projects, especially those that require more flexibility or customization. Developers may need to work within the constraints of Nuxt.js’s conventions or find workarounds for specific requirements.
- Plugin Compatibility: While Nuxt.js has a rich ecosystem of plugins and modules, not all plugins may be compatible with Nuxt.js’s server-side rendering or static site generation features. Developers may need to carefully evaluate plugins and test them in their specific use case.
Nuxt.js Example
<!-- pages/index.vue -->
<template>
<div>
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: "Hello, Nuxt.js!",
};
},
};
</script>
That’s all about Nuxt.js, the Vue.js framework that’s reshaping the way we build modern web applications. With its powerful features, flexible architecture, and delightful developer experience, Nuxt.js continues to empower developers and delight users around the world. So dive in, unleash the magic of Nuxt.js, and elevate your web development journey to new heights!
Nuxt DocumentationConclusion
And there you have it, dear readers – a sidesplitting tour of the most popular JavaScript frameworks in town. Whether you’re a React enthusiast, a Vue aficionado, or a Svelte rebel, there’s a framework out there for every coding style and personality. So go forth, experiment, and remember: in the world of web development, laughter is the best framework of all. Happy coding!