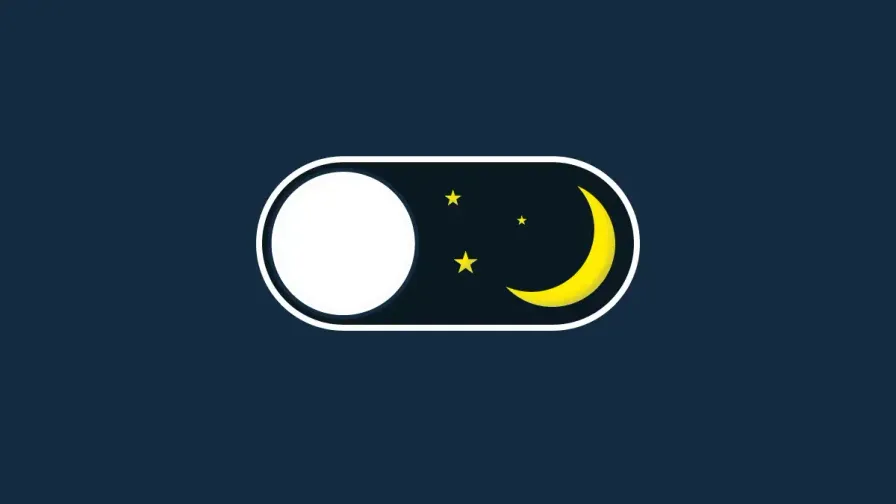
#dark mode
Michał Uzdowski
Implementing dark mode: Adding night vision to your web app
Welcome back to Binary Brain, where we explore the latest trends in web development with a side of humor and a wink to the future. Today, we’re diving into one of the most requested features of modern web applications: Dark Mode. That’s right, folks — the feature that makes your apps look cool, keeps your eyes happy during late-night coding sessions, and gives your battery a bit of extra life.
Dark Mode isn’t just a trend; it’s a lifestyle. It’s the leather jacket of UI design, and everyone wants in on it. But how do you add it to your web application? Is it magic? Witchcraft? An ancient secret passed down by shadowy developers? Nope, it’s just a bit of CSS and JavaScript, and today, I’ll show you exactly how to do it. So, put on your coolest shades and let’s get started!
What is Dark Mode?
Dark Mode is a color scheme that uses light-colored text, icons, and graphical user interface elements on a dark background. It’s not only easier on the eyes during low-light conditions, but it also looks incredibly sleek and modern.
Dark Mode is like the sunglasses of the web world. It’s stylish, makes everything look cooler, and keeps you from squinting like a vampire at sunrise.
Why Add Dark Mode to Your Web Application?
Reduces Eye Strain
Staring at a bright screen all day can make you feel like you’ve been staring directly into the sun. Dark Mode helps reduce eye strain, especially in low-light environments.
Saves Battery Life
On OLED and AMOLED screens, dark pixels use less power. So, implementing Dark Mode can help users save battery life, making them love your app even more.
User Preference
People love customization. Giving users the option to switch to Dark Mode makes them feel in control and enhances their overall experience.
It’s Just Plain Cool
Let’s face it, Dark Mode looks awesome. It’s the difference between wearing a snazzy suit and showing up in sweatpants.
Adding Dark Mode is like turning the lights down low at a party. It instantly makes everything feel cooler, more relaxed, and, let’s be honest, a bit mysterious.
Step-by-Step Guide to Adding Dark Mode
Let’s walk through the process of implementing Dark Mode in your web application, from basic CSS tricks to more advanced techniques involving JavaScript and local storage.
Step 1: Basic Dark Mode with CSS
The simplest way to implement Dark Mode is by using CSS. You can define a set of styles for light mode and another for dark mode.
- Define Your Styles: Start by creating styles for both light and dark modes. Use CSS variables to make toggling between the two modes easier.
/* Define CSS Variables for Light and Dark Themes */
:root {
--background-color: #ffffff;
--text-color: #000000;
}
.dark-mode {
--background-color: #121212;
--text-color: #e0e0e0;
}
/* Apply the Variables */
body {
background-color: var(--background-color);
color: var(--text-color);
transition: background-color 0.3s, color 0.3s;
}
In this example:
:root
defines the default (light) theme..dark-mode
defines the dark theme using CSS variables.- The
body
element uses these variables to set the background and text colors.
- Toggle Dark Mode with JavaScript: Next, add a button that allows users to toggle Dark Mode on and off.
<button id="theme-toggle">Toggle Dark Mode</button>
And add some JavaScript:
// Toggle Dark Mode
document.getElementById("theme-toggle").addEventListener("click", () => {
document.body.classList.toggle("dark-mode");
});
This code adds a click event listener to the button that toggles the dark-mode
class on the body, switching between the light and dark themes.
Think of this toggle button as the light switch in a haunted house. One click, and you’re instantly in the spooky Dark Mode realm.
Step 2: Remember User Preferences with Local Storage
To make your app even more user-friendly, remember the user’s theme preference using local storage. This way, users won’t have to keep switching to Dark Mode every time they visit your site.
- Update Your JavaScript:
// Check for saved user preference, if any, on page load
document.addEventListener("DOMContentLoaded", () => {
const theme = localStorage.getItem("theme");
if (theme === "dark") {
document.body.classList.add("dark-mode");
}
});
// Toggle Dark Mode and save preference
document.getElementById("theme-toggle").addEventListener("click", () => {
document.body.classList.toggle("dark-mode");
const theme = document.body.classList.contains("dark-mode")
? "dark"
: "light";
localStorage.setItem("theme", theme);
});
Now, when users toggle Dark Mode, their preference is saved to local storage, and it’s automatically applied the next time they visit.
This is like your fridge remembering that you prefer soda over water. Every time you open it, your favorite drink is ready and waiting.
Step 3: Use CSS Media Query for System Preferences
Let’s make Dark Mode even smarter by automatically detecting the user’s system preference. Most modern operating systems have a built-in Dark Mode, and you can use a CSS media query to match this preference.
- Add a Media Query to Your CSS:
@media (prefers-color-scheme: dark) {
:root {
--background-color: #121212;
--text-color: #e0e0e0;
}
}
This media query automatically applies the dark theme if the user’s device is set to Dark Mode.
This is like your fridge automatically switching to a beer shelf on Friday nights because it just knows you too well.
Best Practices for Implementing Dark Mode
Keep Contrast in Mind
Make sure there’s enough contrast between the text and background to maintain readability. You don’t want your users squinting at the screen like they’re reading by candlelight.
Use Smooth Transitions
Add a CSS transition to the background and text colors to make the switch between modes smooth and visually pleasing.
body {
transition: background-color 0.3s ease, color 0.3s ease;
}
Test Across Devices
Test Dark Mode on different devices and operating systems to ensure it looks great everywhere. Don’t just rely on your personal setup — Dark Mode can render differently on various screens.
Don’t Forget Images
Ensure your images, icons, and logos look good in both light and dark themes. Transparent PNGs and SVGs work best, but you might need to adjust their colors or use different images based on the theme.
Accessible Colors
Avoid pure black (#000000) and pure white (#FFFFFF). Instead, use off-white and off-black shades to reduce strain on the eyes.
Think of these practices like decorating your house. You want to avoid extreme neon colors that hurt the eyes, make sure the curtains match the carpet, and ensure everything looks cozy in any light.
Advanced Dark Mode Implementation with CSS Variables
Let’s push the boundaries a bit further and explore an advanced implementation using CSS variables. This approach allows for highly customizable themes that can be expanded easily.
- Define CSS Variables for Both Themes:
:root {
--bg-color-light: #ffffff;
--text-color-light: #000000;
--bg-color-dark: #121212;
--text-color-dark: #e0e0e0;
--background-color: var(--bg-color-light);
--text-color: var(--text-color-light);
}
body {
background-color: var(--background-color);
color: var(--text-color);
}
body.dark-mode {
--background-color: var(--bg-color-dark);
--text-color: var(--text-color-dark);
}
In this setup:
- We define light and dark color variables and assign default values.
- When the
dark-mode
class is added to the body, the variable values switch.
- Toggle Themes Using JavaScript:
document.getElementById("theme-toggle").addEventListener("click", () => {
document.body.classList.toggle("dark-mode");
const theme = document.body.classList.contains("dark-mode")
? "dark"
: "light";
localStorage.setItem("theme", theme);
});
This method provides a scalable solution where you can easily adjust or add new variables without rewriting your CSS.
Think of CSS variables like LEGO bricks. You can swap them in and out to build exactly what you need without tearing down the whole structure.
Conclusion
Implementing Dark Mode in your web application is not just a fun feature; it’s becoming a must-have for modern web experiences. With a few lines of CSS and some JavaScript magic, you can offer your users a visually appealing, eye-friendly, and trendy alternative to the usual blinding light theme.
So, whether you’re building a personal blog, a commercial app, or the next big social network, remember that Dark Mode is your ally. It’s the leather jacket of UI design, the coffee of coding environments, and the cool shade on a hot summer’s day.
As always, here at Binary Brain, we make complex things a little less scary and a lot more fun. Happy coding, and may your Dark Mode be ever stylish!