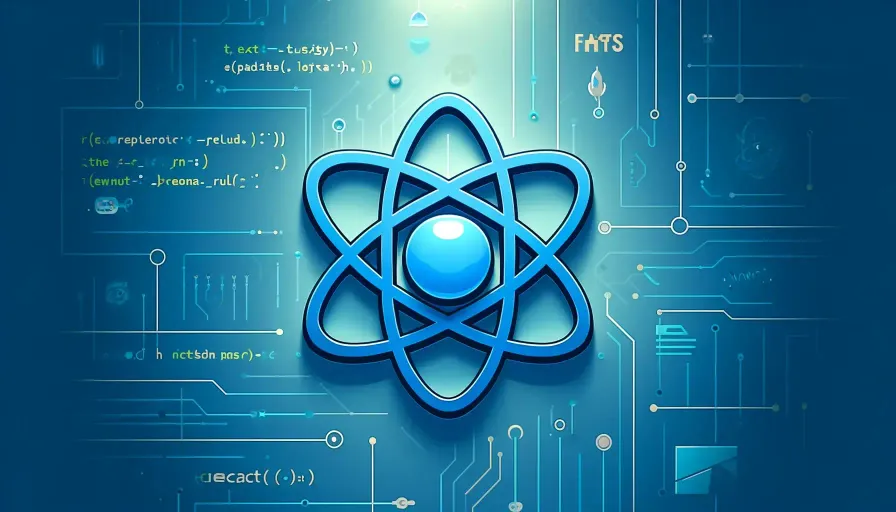
#react
Michał Uzdowski
Building your first React app: A misadventure in coding
Have you ever thought that building a web application is as daunting as cooking a five-star meal for the first time? Well, you’re not alone. Today, we’re going to break down the process of creating your first React application, filled with all the beginner mishaps and some code snippets to save your day — and hopefully, your sanity.
Step 1: Setting Up Your Environment
Before we dive into the deep end, let’s make sure you won’t be coding in a digital swamp. You’ll need Node.js installed on your computer. Think of it as the stove in our five-star kitchen—without it, you’re just chopping vegetables for no reason. Once Node.js is cozy in your system, install Create React App by running in your terminal:
npx create-react-app my-first-react-app
cd my-first-react-app
npm start
Here’s what it looks like: you tell the command line to whip up a new React app called “my-first-react-app”, march into the newly created directory, and kick start the development server. If all goes well, you should see your new React app dancing in your default browser. To follow along, you might want to use the best tools. I have some of them covered in Comprehensive Toolbox for Web Development article.
Step 2: Understanding the Folder Structure
When you open your project, you’ll find that React has scaffolded a bunch of files and folders for you. Here’s a basic breakdown:
- node_modules/: Where all the magic happens — just kidding, it’s where your project’s dependencies live.
- public/: Home of the
index.html
— the canvas of your React masterpiece. - src/: The artist’s studio — where you’ll spend most of your time painting with code.
To help visualize, here’s a simple diagram:
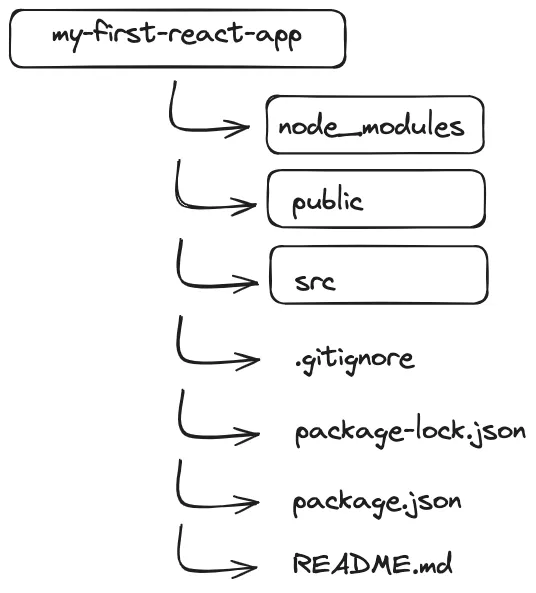
Step 3: Edit src/App.js
Now, to the fun part—coding! Open up src/App.js
. You’ll see some default code that React kindly provides. Let’s tweak it to make our app a bit more personal. Replace the existing code with:
import React from "react";
function App() {
return (
<div>
<h1>Welcome to Binary Brain's React Tutorial!</h1>
<p>
If you're reading this, you've successfully run your first React app.
Isn't that a relief?
</p>
</div>
);
}
export default App;
This code introduces you to JSX, a syntax that looks like HTML but behaves like JavaScript. Confusing? Sure, but less so than trying to understand why cats love boxes. No worries, I’ll explain JSX in a bit.
Step 4: Celebrate and Iterate
After saving your changes, the development server should automatically refresh your browser. Voilà! Your first custom React component is live. Take a moment. Breathe. Celebrate with a cookie or two.
Step 5: Add Some Style
Let’s add a little pizzazz to your app. Find a file called App.css
in the src/
folder and replace it’s content with:
body {
font-family: "Arial", sans-serif;
padding: 30px;
}
h1 {
color: dodgerblue;
}
p {
color: chocolate;
}
Link this CSS file in your App.js
by adding import './App.css';
at the top of the file. This will give your app a bit more character and make it not look like it was designed in the dark ages. You should find your art look something like this:
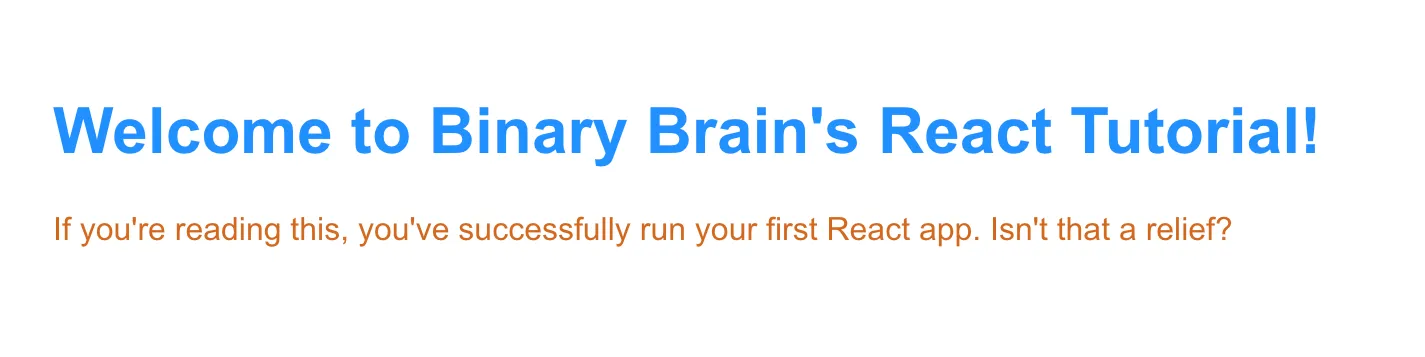
Step 6: Demystifying JSX - React’s Secret Sauce
Now that you’ve dipped your toes into React and have a functioning app, let’s unravel the mystery of JSX, which you’ve already used in App.js
!
JSX stands for JavaScript XML. This is the magical concoction that allows us to write HTML-like syntax directly within JavaScript code. It makes creating UI components more intuitive and readable. But don’t be fooled — under the hood, JSX does a lot more than your typical HTML.
Why Use JSX?
- Readability: JSX code resembles HTML to a great extent, making it easier for developers to understand what the structure will look like.
- Enhanced Productivity: It allows you to visualize and code the UI components at the same time, directly in your JavaScript logic.
- Power of JavaScript: JSX combines the power of JavaScript with HTML like structure, which means you can embed expressions like
{date.toString()}
right inside your JSX structure to dynamically update your UI.
Example of JSX in Action
Let’s expand our App.js
to include a dynamic greeting based on the time of day:
import React from "react";
function App() {
const currentTime = new Date().getHours();
const greeting =
currentTime < 12
? "Good morning"
: currentTime < 18
? "Good afternoon"
: "Good evening";
return (
<div>
<h1>Welcome to Binary Brain's React Tutorial!</h1>
<p>{greeting}, React explorers! You're making great progress.</p>
</div>
);
}
export default App;
In this snippet, we use JavaScript to calculate the current time and determine the appropriate greeting. We then inject this greeting directly into our JSX template in the <p>
tag. This seamless integration of JavaScript and HTML is what makes JSX a powerful tool in React’s arsenal.
How Does JSX Work?
Behind the scenes, JSX is not understood directly by the browsers. It needs to be transpiled into regular JavaScript. This is typically done using a tool like Babel. The JSX:
<div>Hello, world!</div>
is transformed into:
React.createElement("div", null, "Hello, world!");
React uses this object model to efficiently update and render the UI in the web page.
Thinking of JSX as merely ‘HTML in your JavaScript’ simplifies the concept but doesn’t do justice to its power. If you haven’t yet definitely checkout previous articles Mastering HTML and CSS and The Wild World of JavaScript - One Line at a Time. As you become more familiar with React, you’ll appreciate how JSX syntax can greatly enhance the expressiveness and readability of your code, making it easier to develop and maintain your applications.
Wrapping Up
There you have it, a beginner’s guide to building your first app with React, complete with all the steps to make something simple yet satisfying. And fear not, intrepid coder! We’ll dive into the deep waters of more advanced topics like hooks, state management, and other sorceries in future articles. So, keep your life jackets handy—there’s much more to explore in the vast ocean of React. It might seem complex at first, like trying to assemble a spaceship with a toothpick. But with patience and practice, you’ll find it’s more like assembling a really intricate LEGO set — with instructions in Swedish.
Stay tuned for more adventures in coding, and remember, at Binary Brain, we make coding less scary and more fun!