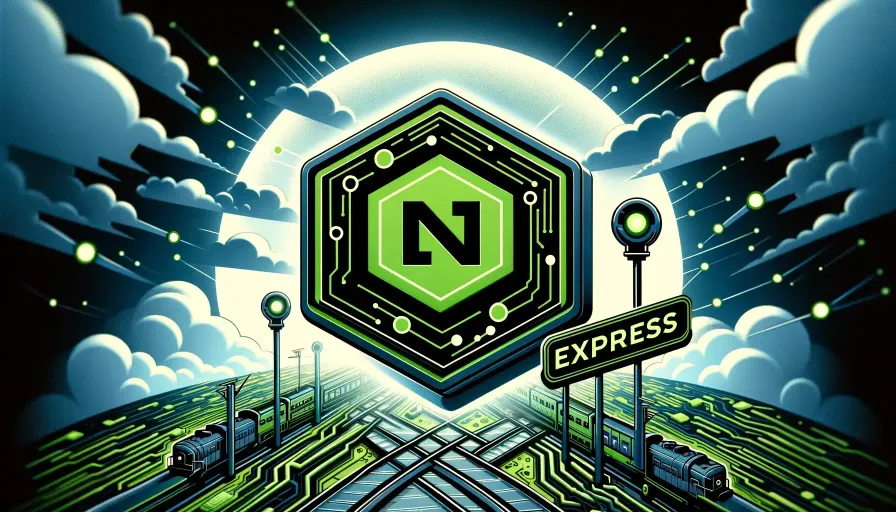
#express
#rest-api
Michał Uzdowski
Building a RESTful API with Node.js and Express: Server yoga for your data
Welcome back to Binary Brain, future API architects!
Today, we’re embarking on a mystical journey to the land of Node.js and Express, where we will master the ancient art of bending servers to our will. Like yoga for your data, we’ll flex, stretch, and position our server to handle requests with the agility of a cat and the stability of a wise old tree. Ready to twist your mind around a RESTful API? Let’s roll out our coding mats and begin.
Step 1: Setting Up Your Node.js Environment
Before we can perform the digital equivalent of a downward dog, we need to set up our Node.js environment. If Node.js isn’t already lounging comfortably on your machine, download and install it from Node.js official website. It’s the yoga mat for our server exercises.
Once Node.js is installed, create a new directory for your project. Open your favorite command line tool, because yes, real developers prefer the command line over GUIs — it’s like preferring black coffee over pumpkin spice lattes. If you haven’t picked your favourite tool yet help yourself with Comprehensive Toolbox for Web Development. Now run:
mkdir my-api-yoga
cd my-api-yoga
npm init -y
This series of commands sets up a new Node.js project. Think of it as your project doing its first stretch of the day.
Step 2: Installing Express
Now, install Express, the minimal and flexible Node.js web application framework. It’s the yoga instructor for our project, guiding it through the various poses and postures of API development.
npm install express
With Express installed, our server is almost ready to bend into all sorts of RESTful positions.
Step 3: Crafting Your First Endpoint
Let’s start with a simple “Hello World” endpoint. This is the API equivalent of reaching your toes without bending your knees. Create a file named app.js
and type in:
const express = require("express");
const app = express();
app.get("/", (req, res) => {
res.send("Hello World!");
});
app.listen(3000, () => {
console.log("API is listening on http://localhost:3000");
});
Let’s break down what’s happening:
require('express')
: This imports the Express library into your file, which is essential for creating the server.
const app = express()
: You’re creating a new instance of an Express application. This is your main object for configuring and starting your web server.
app.get('/')
: Here you define a route. .get refers to HTTP GET requests. The first argument, ’/’, specifies the path, so this route will be triggered when someone navigates to the root URL.
(req, res) => {...}
: This is a callback function that tells the server what to do when the route is matched. req (short for request) is an object containing information about the HTTP request. res (short for response) is an object that lets you craft your HTTP response.
res.send('Hello World!')
: You’re sending the text “Hello World!” back to the client.
app.listen(3000)
: This starts your web server on port 3000, listening for incoming connections.
Run your server with node app.js
and visit http://localhost:3000
. If you see “Hello World!” — congratulations, you’ve just completed your first API asana!
Step 4: Adding More Poses to Your Routine
An API that only says “Hello World” is like a yoga routine with only one pose. Let’s add a CRUD (Create, Read, Update, Delete) functionality to manage items in a todo list:
- Create (POST) - To add new items.
- Read (GET) - To retrieve items.
- Update (PUT) - To modify existing items.
- Delete (DELETE) - To remove items.
Here’s how you’d set this up in Express, expanding our app.js
:
const express = require("express");
const app = express();
// Start with an initial array of to-dos, acting as a pseudo-database for demonstration.
let todos = [{ id: 1, task: "Master Node.js" }];
// READ Operation: Get the list of to-dos.
app.get("/todos", (req, res) => {
res.status(200).json(todos);
});
// CREATE Operation: Add a new to-do to the list.
app.post("/todos", (req, res) => {
const newTodo = { id: todos.length + 1, task: req.body.task };
todos.push(newTodo);
res.status(201).send(newTodo);
});
// UPDATE Operation: Modify an existing to-do.
app.put("/todos/:id", (req, res) => {
let todo = todos.find((t) => t.id === parseInt(req.params.id));
if (!todo) res.status(404).send("The todo with the given ID was not found");
todo.task = req.body.task;
res.send(todo);
});
// DELETE Operation: Remove a to-do from the list.
app.delete("/todos/:id", (req, res) => {
const originalTodosLength = todos.length;
todos = todos.filter((t) => t.id !== parseInt(req.params.id));
if (todos.length === originalTodosLength)
res.status(404).send("The todo with the given ID was not found");
res.send(todos);
});
app.listen(3000, () => {
console.log("API is listening on http://localhost:3000");
});
Each of these route handlers is set up to perform a specific action on the server when a request is made:
READ app.get('/todos')
: When a GET request is sent to /todos, the server responds with the entire list of to-dos in JSON format. It’s the server’s way of saying, “Here’s what you need to get done!”
CREATE app.post('/todos')
: POST requests to /todos expect a new task in the request body. This operation takes that task, creates a new to-do item, adds it to the list, and returns the new item as confirmation. It’s like saying, “Got it, adding this to your list!”
UPDATE app.put('/todos/:id')
: PUT requests to /todos/:id are for updating an existing to-do. The :id is a placeholder for the specific to-do’s id. The code finds the to-do by this id, updates it, and sends back the updated item. It’s the API’s version of, “Alright, changing plans!”
DELETE app.delete('/todos/:id')
: DELETE requests to /todos/:id tell the server to remove the to-do with the given id. If the to-do exists, it’s filtered out, and the server returns the updated list, confirming the removal with a (kind of) “Done and dusted, it’s off your list!”
This CRUD setup is like teaching your server different yoga poses. Each pose (or operation) allows the server to interact with the data (or to-do list) in a specific and useful way, making your API flexible and responsive to the needs of the users.
Step 5: Testing Your Flexibility
Test your API using a tool like Postman. It’s like having a mirror in front of you while you practice your poses. Make sure each endpoint is flexing correctly and responding as expected.
Example API request with Postman
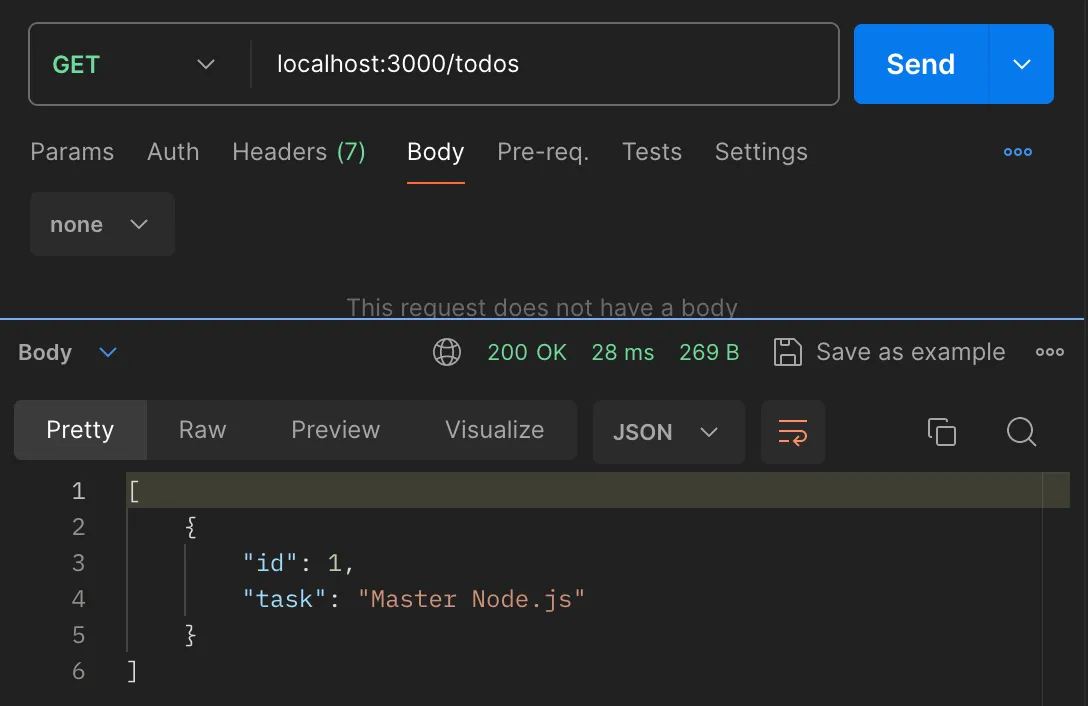
Wrapping Up
There you have it! A RESTful API with Node.js and Express, ready to handle any client requests with the grace of a seasoned yoga instructor. Just as yoga keeps your body healthy and flexible, a well-designed API keeps your application functional and efficient.
Stay tuned for more tech adventures, and remember, at Binary Brain, we turn complicated into simple and add a pinch of fun to coding! Happy developing!