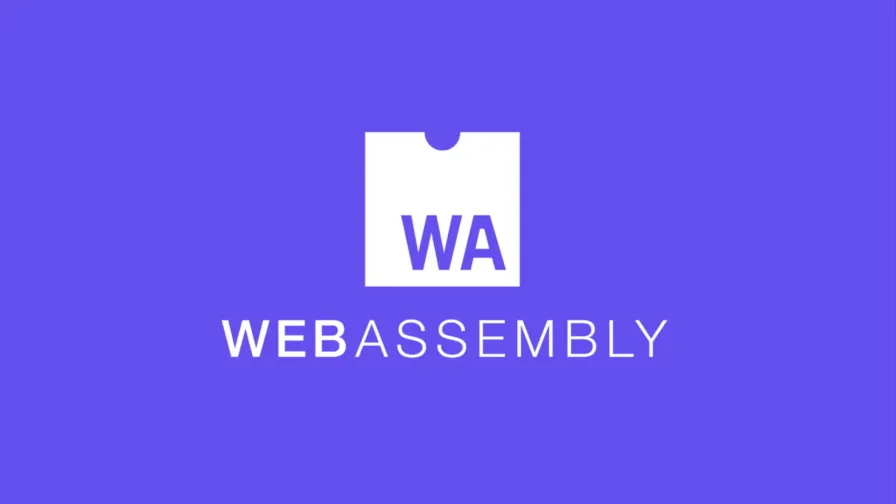
#architecture
Michał Uzdowski
Demystifying WebAssembly: The turbo boost your web apps didn’t know they neded
Welcome back to Binary Brain, the tech blog where we break down complex concepts with humor, wisdom, and enough geekiness to make even your code crack a smile. Today, we’re tackling a subject that’s been quietly revolutionizing web performance: WebAssembly.
You may have heard whispers about WebAssembly (Wasm), but much like the mysterious Bermuda Triangle or why your cat insists on sitting on your laptop, it can seem a bit puzzling at first. What is this WebAssembly magic? Is it sorcery? A tool only reserved for elite developers? Or is it the secret sauce to turning your sluggish web app into a Formula 1 racer?
Spoiler alert: It’s none of those things. WebAssembly is a binary instruction format that allows code to run at near-native speeds, right in the browser. In simpler terms: it makes your web apps fast — like, “hold onto your keyboards” fast.
So, let’s pull back the curtain and take a closer look at what WebAssembly is, how it works, and why it could be the performance boost your web applications have been dreaming about. And, of course, we’ll throw in some humor along the way to make things fun.
What is WebAssembly (Wasm)?
Before we dive into the how, let’s start with the what.
WebAssembly (Wasm) is a low-level, binary instruction format that allows you to run code written in languages like C, C++, Rust, and others at near-native speed directly in the browser. It was designed to be a companion to JavaScript, not a replacement. In fact, WebAssembly and JavaScript often work hand-in-hand to create high-performance web applications.
In practical terms, WebAssembly allows developers to write parts of their web application in languages other than JavaScript (gasp!), compile them into WebAssembly, and then execute them in the browser. The result? Code that runs almost as fast as if it were natively installed on your computer.
Imagine JavaScript is your trusty bicycle. It’s reliable, it gets the job done, but it’s not breaking any speed records. WebAssembly is like strapping a jet engine to that bicycle. Now, you’re not just cruising around the neighborhood — you’re flying down the highway at breakneck speeds!
Why WebAssembly? The Need for Speed
Why should you care about WebAssembly? Here’s the deal: while JavaScript is great for building interactive web applications, it’s not always the fastest kid on the block. For tasks that require heavy computation — like video processing, image manipulation, or running complex algorithms — JavaScript can struggle to keep up.
Enter WebAssembly. By running at near-native speeds, WebAssembly opens the door to a whole new world of web development possibilities. Think:
- Real-time video editing in the browser
- High-performance 3D games
- Machine learning models running directly on the client
- Faster data analysis tools
JavaScript is like cooking a pizza in your regular kitchen oven. It’s fine, it works, but it’s not exactly a wood-fired pizza oven that cooks the perfect pie in two minutes flat. WebAssembly is that wood-fired oven, giving you delicious performance faster than you thought possible.
How Does WebAssembly Work?
Now that we know why WebAssembly is amazing, let’s dive into the how. How does WebAssembly work its magic?
Compiling to WebAssembly
The first step in the WebAssembly process is writing your code in a compiled language like C, C++, Rust, or AssemblyScript (a TypeScript-like language for WebAssembly). Once your code is written, you compile it into a binary format that the browser can understand — this is the WebAssembly (Wasm) module.
Here’s a basic example of how you’d compile a C program to WebAssembly:
emcc hello.c -o hello.wasm
In this example, the emcc command is part of Emscripten, a tool that compiles C and C++ code into WebAssembly. After running this, you’ll have a .wasm
file that your web app can load and execute.
Running WebAssembly in the Browser
Once your code is compiled into a .wasm
file, it can be loaded and executed by the browser. WebAssembly is supported in all major browsers, including Chrome, Firefox, Safari, and Edge.
Here’s a simple example of how you’d load and run a WebAssembly module in JavaScript:
fetch("hello.wasm")
.then((response) => response.arrayBuffer())
.then((bytes) => WebAssembly.instantiate(bytes))
.then((results) => {
console.log(results.instance.exports.main());
});
This code fetches the .wasm
file, instantiates it, and then runs the main function exported from the WebAssembly module. And boom — your WebAssembly code is now running in the browser at blazing speed.
It’s like sending your JavaScript code to a high-intensity boot camp. It comes back as WebAssembly: stronger, faster, and ready to take on whatever you throw at it — whether it’s crunching numbers or rendering graphics in real-time.
WebAssembly + JavaScript: The Dynamic Duo
Here’s the important thing to remember: WebAssembly isn’t here to replace JavaScript. It’s a partner, not a competitor. JavaScript is still incredibly flexible and easy to work with, and it excels at handling UI updates, DOM manipulation, and event handling.
WebAssembly, on the other hand, shines when it comes to performance-heavy tasks — things like complex calculations, cryptography, and image processing. In fact, the best web apps of the future will likely use both JavaScript and WebAssembly, with each handling the tasks they’re best suited for.
Example: Imagine you’re building a web-based image editor. You could use JavaScript for the user interface, allowing users to drag and drop images, apply filters, and save their work. But when it comes to heavy-duty image processing (like resizing a 4K image in real time), you’d offload that task to WebAssembly.
It’s a perfect balance of speed and flexibility.
Think of WebAssembly and JavaScript like Batman and Robin. JavaScript is the all-around hero, great at many things, but sometimes needs a little help when the heavy lifting comes in. That’s where WebAssembly (Robin) swoops in with some supercharged backup.
Use Cases for WebAssembly: When Should You Use It?
You might be wondering: “Sure, WebAssembly sounds cool, but when should I actually use it?” Here are some prime use cases where WebAssembly can really shine:
Gaming in the Browser
WebAssembly is perfect for running high-performance 3D games in the browser. By combining WebGL for rendering and WebAssembly for the heavy computation, you can create games that run at near-native speeds without requiring users to download any software.
Example: The popular game engine Unity can compile games into WebAssembly, allowing them to run directly in the browser. Now, you can play high-quality games without installing anything — right from the comfort of your browser tab.
Video Editing
Video processing is one of the most computationally intensive tasks out there, and doing it in JavaScript can be, well, painful. WebAssembly allows you to perform real-time video encoding, filtering, and editing right in the browser.
Example: Projects like FFmpeg have been compiled into WebAssembly, enabling video manipulation directly in the browser. This is a game-changer for apps that require real-time video editing or live streaming.
Machine Learning
Machine learning requires a lot of computational power, and WebAssembly can help bring machine learning models directly to the browser, allowing for real-time inference without needing to rely on backend servers.
Example: Libraries like TensorFlow.js have started using WebAssembly to accelerate machine learning tasks in the browser. This opens up the possibility of building web apps that can run complex AI models in real time without requiring heavy server infrastructure.
Cryptography
Cryptography algorithms are notoriously expensive to run. WebAssembly can be used to speed up encryption, decryption, and hashing processes, making secure web applications more efficient.
Example: If you’re building an app that requires heavy encryption (say, a secure messaging app), WebAssembly can handle the cryptographic functions much faster than JavaScript alone.
How to Get Started with WebAssembly
Ready to jump into WebAssembly? Here’s how you can get started:
Pick a Language
Choose a language that compiles to WebAssembly, such as C, C++, Rust, or AssemblyScript. Rust, in particular, has become very popular in the WebAssembly world due to its performance and safety features.
Install the Toolchain
For C and C++, you can use Emscripten to compile your code into WebAssembly. For Rust, you can use the wasm-pack tool to get started quickly.
Write Your Code
Write the performance-critical parts of your app in the chosen language and compile them into WebAssembly.
Integrate with JavaScript
Use JavaScript to load and execute your WebAssembly modules, integrating them with the rest of your app.
Example: Here’s a super-simple Rust example:
// lib.rs
#[no_mangle]
pub fn add
(a: i32, b: i32) -> i32 {
a + b
}
After compiling this Rust code into WebAssembly, you can load and call the add
function from JavaScript, using WebAssembly’s super speed to perform the operation.
Challenges of WebAssembly
No technology is without its challenges, and WebAssembly is no exception. Here are a few things to keep in mind:
Limited Browser API Access
WebAssembly doesn’t have direct access to the DOM or certain web APIs, meaning you’ll still need to rely on JavaScript for things like event handling and DOM manipulation.
Debugging Can Be Tricky
Debugging WebAssembly code isn’t as straightforward as debugging JavaScript. You often have to debug the source language (like C or Rust) and then map that back to the WebAssembly binary.
Learning Curve
If you’re only familiar with JavaScript, there’s a bit of a learning curve to pick up languages like Rust or C and learn how to compile them to WebAssembly.
It’s like learning to drive a sports car after years of driving a compact sedan. It’s fast, it’s fun, but if you’re not careful, you might hit a few bumps along the way.
Conclusion
WebAssembly is a game-changing technology that brings near-native performance to web applications. By allowing developers to write high-performance code in languages like C, C++, and Rust, and then run that code in the browser, WebAssembly opens the door to a new era of web development.
Whether you’re building a high-performance game, a video editing app, or a machine learning-powered tool, WebAssembly can give you the performance boost you need.
And remember — WebAssembly and JavaScript work best when used together. JavaScript handles the flexibility and user interaction, while WebAssembly tackles the performance-heavy tasks. Together, they create the ultimate dynamic duo for modern web development.
So, what are you waiting for? Give your web apps the turbo boost they deserve and start exploring WebAssembly today. And as always, here at Binary Brain, we’re here to guide you through the complexities with humor, tips, and a few laughs along the way.
Happy coding, and may your apps be as fast as a Wasm rocket! 🚀