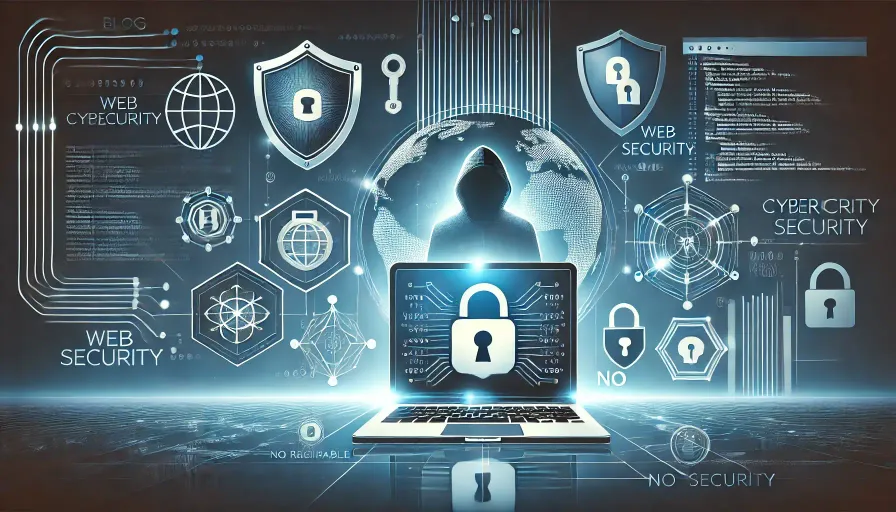
#cybersecurity
Michał Uzdowski
Cybersecurity practices in web development: Locking down your code with style
Welcome back to Binary Brain, fellow developers and digital security aficionados! Today, we’re diving into the wild world of cybersecurity in web development. In a realm where the only thing hackers love more than your personal data is finding new ways to steal it, it’s crucial to lock down your code with style. Think of this as your ultimate guide to becoming the James Bond of web security — minus the tuxedo, but with all the cool gadgets.
Why Cybersecurity Matters
Before we get into the nitty-gritty of securing your web applications, let’s talk about why cybersecurity is so important. Imagine building a beautiful, modern house but leaving all the doors and windows wide open. Not exactly smart, right? That’s what it’s like having a slick website without proper security measures. Cyberattacks can lead to data breaches, financial loss, and a tarnished reputation.
Cybersecurity is not just about protecting data; it’s about ensuring the integrity, confidentiality, and availability of your web applications. Now, let’s arm ourselves with some top-notch security practices.
Top Cybersecurity Practices for Web Development
1. HTTPS Everywhere
Enforcing HTTPS not only encrypts data in transit but also boosts your site’s SEO ranking. Search engines like Google give preference to secure sites. Additionally, it builds user trust, making visitors more likely to interact with your website and share personal information.
Why: HTTPS encrypts data between the user’s browser and your server, protecting sensitive information from eavesdroppers.
How: Obtain an SSL/TLS certificate from a trusted Certificate Authority (CA) and configure your server to enforce HTTPS. Many hosting providers offer free SSL certificates through Let’s Encrypt.
Pro Tip: Redirect all HTTP traffic to HTTPS to ensure all communications are secure.
# Example Nginx configuration to redirect HTTP to HTTPS
server {
listen 80;
server_name example.com;
return 301 https://$host$request_uri;
}
2. Secure Authentication
Ensure you have account lockout mechanisms to prevent brute-force attacks. Also, consider implementing passwordless authentication using technologies like WebAuthn, which can enhance security and user experience by eliminating the need for traditional passwords.
Why: Authentication is the gateway to your application. Weak authentication methods can lead to unauthorized access.
How: Use multi-factor authentication (MFA) and strong password policies. Implement OAuth or OpenID Connect for secure third-party authentication.
Pro Tip: Never store plain text passwords. Always hash passwords using a strong algorithm like bcrypt.
// Example using bcrypt to hash a password in Node.js
const bcrypt = require("bcrypt");
const saltRounds = 10;
const plainTextPassword = "mySuperSecretPassword";
bcrypt.hash(plainTextPassword, saltRounds, function (err, hash) {
// Store hash in your database
});
3. Input Validation and Sanitization
Besides validation, employ techniques like parameterized queries and prepared statements to prevent SQL injection. For XSS protection, utilize output encoding to escape user input before rendering it on the page.
Why: User inputs are a common attack vector for SQL injection, XSS, and other attacks.
How: Validate and sanitize all user inputs on both the client and server sides. Use libraries like Joi for input validation.
Pro Tip: Never trust user inputs. Assume they are trying to hack your system until proven otherwise.
// Example using Joi for input validation in Node.js
const Joi = require("joi");
const schema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
password: Joi.string().pattern(new RegExp("^[a-zA-Z0-9]{3,30}$")).required(),
});
const { error, value } = schema.validate({
username: "abc",
password: "123",
});
if (error) {
console.error("Validation error:", error.details);
}
4. Use Security Headers
Security headers also include Referrer-Policy to control how much referrer information is shared and Feature-Policy to enable or disable certain browser features like geolocation or microphone access. Setting these headers appropriately can greatly enhance the security posture of your web application.
Why: Security headers protect your application from a range of common vulnerabilities by setting rules in HTTP responses.
How: Configure your server to include headers like Content-Security-Policy (CSP), X-Content-Type-Options, X-Frame-Options, and Strict-Transport-Security (HSTS).
Pro Tip: Use a library like Helmet in Express.js to set security headers easily.
// Example using Helmet in an Express.js app
const helmet = require("helmet");
const express = require("express");
const app = express();
app.use(helmet());
5. Regular Security Audits and Penetration Testing
Besides automated tools, engage with professional penetration testers who can provide a more thorough assessment by thinking like a hacker. Incorporate security audits into your continuous integration pipeline to catch issues early in the development process.
Why: Regular security audits help identify and fix vulnerabilities before attackers exploit them.
How: Perform regular code reviews, use automated security scanners, and conduct penetration tests.
Pro Tip: Consider using services like OWASP ZAP or Burp Suite for comprehensive security testing.
# Running OWASP ZAP in Docker for automated security scanning
docker run -u zap -p 8080:8080 owasp/zap2docker-stable zap-webswing.sh
6. Secure APIs
Ensure your API only allows HTTPS connections. Implement fine-grained access controls with role-based access control (RBAC) to restrict what each user can do. Use JSON Web Tokens (JWT) for securely transmitting information between parties as a JSON object.
Why: APIs are a prime target for attackers. Securing them is critical to protecting your data and services.
How: Use strong authentication and authorization, validate inputs, and implement rate limiting to prevent abuse.
Pro Tip: Use API gateways like Kong or AWS API Gateway to manage and secure your APIs.
# Example AWS API Gateway configuration for rate limiting
resources:
Resources:
ApiGatewayRestApi:
Type: "AWS::ApiGateway::RestApi"
Properties:
Name: "MyApi"
UsagePlan:
Type: "AWS::ApiGateway::UsagePlan"
Properties:
Throttle:
BurstLimit: 100
RateLimit: 50
7. Secure Data Storage
Besides encryption, ensure proper data backup strategies are in place. Use database encryption features like Transparent Data Encryption (TDE) for SQL databases. For file storage, encrypt individual files and manage access permissions strictly.
Why: Protecting stored data is as important as protecting data in transit.
How: Encrypt sensitive data at rest using strong encryption algorithms like AES-256.
Pro Tip: Regularly rotate encryption keys and store them securely using key management services like AWS KMS or Azure Key Vault.
# Example using AWS KMS to encrypt data
aws kms encrypt --key-id alias/my-key --plaintext fileb://data.txt --output text --query CiphertextBlob
8. Implement Logging and Monitoring
Ensure logs are immutable and protected from tampering. Set up alerts for anomalies such as multiple failed login attempts or unusual data access patterns. Regularly review logs and use machine learning to detect potential security threats.
Why: Monitoring your application helps detect and respond to security incidents in real-time.
How: Implement logging for all critical actions and use monitoring tools to track suspicious activities.
Pro Tip: Use services like Splunk, ELK Stack, or AWS CloudWatch for centralized logging and monitoring.
# Example ELK Stack configuration for logging
filebeat.inputs:
- type: log
paths:
- /var/log/*.log
output.elasticsearch:
hosts: ["http://localhost:9200"]
9. Educate Your Team
Implement a security champion program within your development team where certain members are designated as security experts. Encourage regular knowledge sharing and create a culture of security awareness. Use interactive training platforms like KnowBe4 for engaging cybersecurity training.
Why: Security is a team effort. Ensuring that everyone is aware of best practices helps maintain a secure development environment.
How: Conduct regular training sessions and workshops on cybersecurity.
Pro Tip: Use resources like OWASP Top Ten and SANS Institute to keep your team informed about the latest threats and defenses.
10. Secure Development Lifecycle (SDLC)
Incorporate security checkpoints in your SDLC stages, from planning to deployment. Use threat modeling to identify and mitigate potential risks early. Regularly update and patch all software dependencies to fix known vulnerabilities.
Why: Integrating security into every phase of the development lifecycle helps catch vulnerabilities early.
How: Adopt practices like threat modeling, static code analysis, and automated security testing.
Pro Tip: Use tools like SonarQube for static code analysis and Jenkins for continuous integration and deployment (CI/CD) pipelines.
// Example Jenkins pipeline with SonarQube integration
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sonarScanner(
'SonarQube Scanner for Jenkins',
'sonar-project.properties'
)
}
}
}
}
}
Conclusion
And there you have it, folks — your guide to becoming a web development security superstar! Adopting these cybersecurity practices will not only help protect your web applications from the bad guys but also give you peace of mind knowing that your data and your users are safe.
Remember, cybersecurity is not a one-time task but an ongoing process. Stay informed, stay vigilant, and keep your code locked down tight. And as always, stay tuned to Binary Brain for more tech adventures with a twist of humor. Happy coding, and may your security be unbreakable!