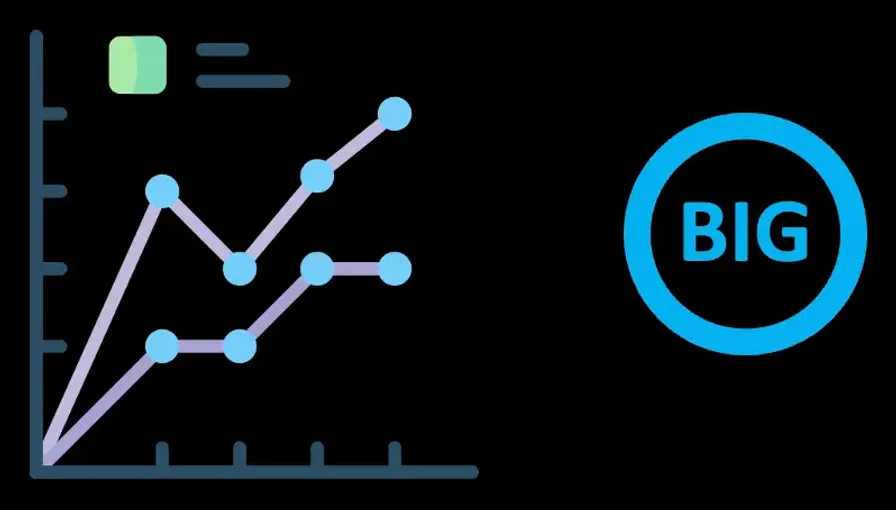
#big-o
#algorithms
Michał Uzdowski
Understanding Big O notation: The secret language of algorithms
Welcome back to Binary Brain, where we unravel the mysteries of the tech world with a dash of humor and a sprinkle of wit. Today, we’re diving into the magical, often misunderstood world of Big O Notation — the secret language of algorithms that sounds like something a wizard might chant before casting a spell. If you’ve ever been puzzled by those cryptic expressions like O(1), O(n), or, heaven forbid, O(n²), then you’re in the right place.
Big O Notation isn’t just for computer scientists in ivory towers; it’s a crucial concept for every developer. Whether you’re optimizing your code to run faster than your coffee machine in the morning or just trying to sound smart in meetings, understanding Big O is key. So, let’s don our wizard hats and start making sense of this mystical math!
What is Big O Notation?
Big O Notation is a way to describe how the performance of an algorithm changes as the size of the input grows. It helps you understand the efficiency of your code by focusing on how it scales, rather than just how fast it runs on your laptop. Big O tells you whether your algorithm will handle millions of users or crash like a Windows 98 PC with too many browser tabs open.
Imagine you’re planning a party. Big O Notation is like figuring out how much pizza you’ll need as the guest list grows. If you’re just having a couple of friends over, you’re fine with one large pizza (O(1)). But if you’re inviting the whole town, you better start calculating some serious pizza logistics (O(n²)).
Why is Big O Important?
Big O is important because it gives you a way to predict how your code will perform as your data scales. Here’s why you should care:
- Performance Optimization: Big O helps you identify bottlenecks in your code and make smarter decisions when choosing algorithms.
- Scalability: Writing code that works on small datasets is one thing; making sure it scales efficiently to handle large amounts of data is another.
- Interview Prep: Big O is a staple of technical interviews. If you can talk Big O, you’re already halfway to acing that coding challenge.
Think of Big O as the weather forecast for your code. If you know a storm of data is coming, Big O tells you whether your algorithm is going to weather it like a champ or collapse like an umbrella in a hurricane.
Common Big O Notations
Let’s explore the most common Big O notations you’ll encounter, from the delightfully efficient to the dreadfully slow.
O(1) - Constant Time
Description: O(1) means that the time it takes to run your algorithm is constant, no matter how much data you have. It’s the gold standard of efficiency.
Example: Accessing an element in an array by its index.
function getFirstElement(array) {
return array[0]; // No matter the size of the array, this operation takes the same amount of time.
}
O(1) is like grabbing a soda from your fridge — it doesn’t matter how full the fridge is, it takes the same amount of time to reach in and grab a drink.
O(log n) - Logarithmic Time
Description: O(log n) algorithms reduce the problem size by half each time, making them super efficient for large datasets. This is common in algorithms like Binary Search.
Example: Binary Search.
function binarySearch(sortedArray, target) {
let left = 0;
let right = sortedArray.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (sortedArray[mid] === target) {
return mid; // Found the target
} else if (sortedArray[mid] < target) {
left = mid + 1; // Narrow the search to the right half
} else {
right = mid - 1; // Narrow the search to the left half
}
}
return -1; // Target not found
}
O(log n) is like finding a name in a phone book (remember those?). You don’t start at the first page — you open to the middle, see where you are, and then jump forward or backward, halving the search space each time.
O(n) - Linear Time
Description: O(n) means the time it takes grows linearly with the size of the input. If you double the data, you double the time.
Example: Looping through an array.
function printAllElements(array) {
for (let i = 0; i < array.length; i++) {
console.log(array[i]);
}
}
O(n) is like waiting in line at the grocery store. The more people in front of you, the longer it takes. No special tricks — just patience.
O(n log n) - Linearithmic Time
Description: O(n log n) is often seen in efficient sorting algorithms like Merge Sort and Quick Sort. It’s faster than O(n²) but not as slick as O(n).
Example: Merge Sort.
function mergeSort(array) {
if (array.length < 2) return array;
const mid = Math.floor(array.length / 2);
const left = mergeSort(array.slice(0, mid));
const right = mergeSort(array.slice(mid));
return merge(left, right);
}
function merge(left, right) {
let sortedArray = [];
while (left.length && right.length) {
if (left[0] <= right[0]) {
sortedArray.push(left.shift());
} else {
sortedArray.push(right.shift());
}
}
return [...sortedArray, ...left, ...right];
}
O(n log n) is like sorting your laundry. You break it into smaller piles, sort each pile, and then merge them back together in order. It’s more efficient than sorting the whole mess at once.
O(n²) - Quadratic Time
Description: O(n²) means the time it takes grows quadratically as the input size grows. It’s often seen in algorithms with nested loops, like Bubble Sort.
Example: Bubble Sort.
function bubbleSort(array) {
for (let i = 0; i < array.length; i++) {
for (let j = 0; j < array.length - i - 1; j++) {
if (array[j] > array[j + 1]) {
[array[j], array[j + 1]] = [array[j + 1], array[j]];
}
}
}
return array;
}
O(n²) is like planning a wedding seating chart where every guest has opinions about every other guest. The more guests you have, the more complicated (and annoying) it gets.
O(2ⁿ) - Exponential Time
Description: O(2ⁿ) means the time it takes doubles with each additional input. This is typical of recursive algorithms that solve problems by breaking them into multiple smaller sub-problems.
Example: Solving the Fibonacci sequence recursively.
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
O(2ⁿ) is like breeding rabbits. You start with one pair, and suddenly you’re overwhelmed with bunnies. Every step doubles the population, and before you know it, you’re knee-deep in rabbits (or recursive calls).
O(n!) - Factorial Time
Description: O(n!) is the worst of the worst, often seen in algorithms that try every possible combination, like solving the Traveling Salesman Problem through brute force.
Example: Generating all permutations of a string.
function permute(str) {
if (str.length <= 1) return [str];
const perms = [];
for (let i = 0; i < str.length; i++) {
const char = str[i];
const remaining = str.slice(0, i) + str.slice(i + 1);
for (let perm of permute(remaining)) {
perms.push(char + perm);
}
}
return perms;
}
O(n!) is like being given a deck of cards and being told to find every possible way to shuffle them. With 52 cards, you’ve got more combinations than atoms in the observable universe. Good luck with that!
How to Analyze Algorithms with Big O
Analyzing algorithms with Big O notation is about finding the worst-case scenario and focusing on the most significant factors that affect performance. Here’s a quick guide:
-
Drop the Constants: If your algorithm runs in O(2n) time, just call it O(n). We care about how things scale, not the exact coefficients.
-
Focus on the Biggest Term: In O(n + n²), the n² term dominates as the input size grows, so we simplify it to O(n²).
-
Look for Nested Loops: Each level of nesting usually means multiplying the Big O by another factor of n.
Analyzing Big O is like reading a recipe. Focus on the longest cooking step because that’s what’s going to hold up dinner, not the two seconds it takes to sprinkle some salt.
Conclusion
Big O Notation is a powerful tool that helps you understand and communicate the efficiency of your algorithms. From O(1) to O(n!), each notation tells a different story about how your code scales — and whether it’s fit for the job or doomed to a slow, painful demise.
So, the next time someone asks you about the efficiency of your code, don’t just shrug and say, “It works fine on my machine”. Instead, proudly bust out some Big O lingo, and watch as everyone nods in impressed confusion.
As always, keep coding, keep learning, and remember: Here at Binary Brain, we make even the scariest topics a little less terrifying and a whole lot more fun. Happy coding!