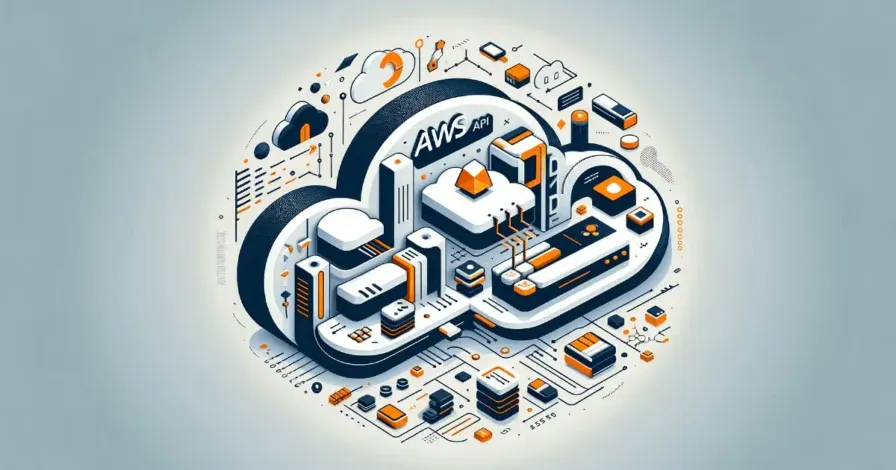
#serverless
#aws
#api
Michał Uzdowski
Building scalable APIs with API Gateway and Lambda: Serverless nirvana
Welcome back to Binary Brain, where we turn complex tech concepts into simple, digestible, and sometimes downright hilarious reads. Today, we’re diving into the world of building scalable APIs using API Gateway and Lambda. Imagine creating a robust, scalable backend without ever touching a server. Sounds like a dream, right? Well, buckle up, because we’re about to make that dream a reality!
What Are API Gateway and Lambda?
Before we dive into the nitty-gritty, let’s get to know our main characters:
API Gateway
Think of it as the bouncer at the club, managing who gets in and what they can do. It sits at the front of your API, handling all the incoming requests and directing them to the appropriate place.
AWS Lambda
This is the star of the show, performing tasks whenever called upon. It’s a serverless compute service that lets you run code without provisioning or managing servers. You write the code, upload it, and Lambda takes care of the rest.
Why Use API Gateway and Lambda?
Scalability
These services are designed to scale automatically with your traffic. Whether you’re handling ten requests per day or ten thousand per second, they can handle it.
AWS Lambda scales out by running your code in response to each trigger. API Gateway, on the other hand, can manage thousands of concurrent API calls.
Imagine having a pizza place that magically hires more chefs as more orders come in, ensuring no one ever has to wait. That’s API Gateway and Lambda for you.
Cost-Effectiveness
You only pay for what you use. There’s no need to keep a server running 24/7.
Lambda charges you based on the number of requests and the duration your code runs. API Gateway pricing is based on the number of calls to your APIs.
It’s like only paying for your pizza when you’re actually hungry and ordering, rather than having a chef on standby all day.
Ease of Maintenance
No servers to manage means less headache.
AWS handles the infrastructure, scaling, and maintenance. You focus on your code.
Feels like having a self-cleaning kitchen. You just cook, and the mess disappears on its own.
Setting Up Your Environment
Let’s get our hands dirty. We’ll set up a basic environment to build and deploy a scalable API.
Step 1: Setting Up AWS CLI
First things first, if you haven’t already, install and configure the AWS Command Line Interface (CLI). This will allow you to interact with AWS services from your terminal.
npm install -g awscli
aws configure
You’ll need your AWS Access Key ID, Secret Access Key, region, and output format. If you don’t have these, head over to the AWS Management Console to create them.
Step 2: Creating a Simple Lambda Function
Next, let’s create a simple Lambda function. For this example, we’ll write a function that returns a list of todos.
index.js:
exports.handler = async (event) => {
const todos = [
{ id: 1, task: "Learn AWS Lambda" },
{ id: 2, task: "Set up API Gateway" },
{ id: 3, task: "Build a scalable API" },
];
return {
statusCode: 200,
body: JSON.stringify(todos),
};
};
Zip this file to prepare it for upload:
zip function.zip index.js
Now, create the Lambda function using the AWS CLI:
aws lambda create-function \
--function-name GetTodos \
--zip-file fileb://function.zip \
--handler index.handler \
--runtime nodejs14.x \
--role arn:aws:iam::YOUR_ACCOUNT_ID:role/YOUR_LAMBDA_ROLE
Make sure to replace YOUR_ACCOUNT_ID
and YOUR_LAMBDA_ROLE
with your actual AWS account ID and IAM role ARN.
Step 3: Setting Up API Gateway
Now that we have our Lambda function, let’s create an API Gateway to expose it as a RESTful API.
-
Create a new API
- Go to the API Gateway Console.
- Click on “Create API”.
- Choose “REST API” and click “Build”.
-
Configure the API
- Give your API a name (e.g., “TodosAPI”).
- Click “Create API”.
-
Create a Resource
- Under your new API, click “Resources”.
- Click “Actions” > “Create Resource”.
- Enter “todos” as the Resource Name and Resource Path.
- Click “Create Resource”.
-
Create a Method
- Select the
/todos
resource. - Click “Actions” > “Create Method”.
- Choose “GET” from the dropdown and click the checkmark.
- Select the
-
Integrate with Lambda
- Select “Lambda Function” as the integration type.
- Check “Use Lambda Proxy integration”.
- Enter the region and name of your Lambda function (e.g.,
GetTodos
). - Click “Save”.
-
Deploy the API
- Click “Actions” > “Deploy API”.
- Create a new stage (e.g., “prod”).
- Click “Deploy”.
You will receive a URL for your API endpoint. Open it in your browser, and you should see the list of todos returned by your Lambda function.
Step 4: Testing and Scaling
Now that your API is up and running, let’s test it and see how it scales.
Testing
Use a tool like Postman (read The Power of Postman: A Comprehensive Tool for API Development if you haven’t mastered it yet) or curl
to test your API endpoint.
curl https://YOUR_API_ID.execute-api.YOUR_REGION.amazonaws.com/prod/todos
You should get a JSON response with the list of todos.
Scaling
AWS Lambda and API Gateway handle scaling automatically. As your traffic increases, AWS will handle the load by spinning up more instances of your Lambda function. You don’t have to worry about manually scaling or maintaining servers.
Advanced Features and Best Practices
Once you have the basics down, it’s time to explore some advanced features and best practices to make your API even more powerful and efficient.
Adding Authentication
Protect your API endpoints from unauthorized access.
Use AWS IAM roles, API keys, or Amazon Cognito to manage authentication.
Amazon Cognito is a great option for adding authentication to your API. It allows you to create user pools and identity pools to manage user authentication and authorization. You can also integrate it with third-party identity providers like Google, Facebook, and Amazon.
Monitoring and Logging
Keep track of your API’s performance and diagnose issues quickly.
Use AWS CloudWatch to monitor your Lambda functions and API Gateway.
CloudWatch provides detailed metrics on the performance of your Lambda functions, including invocation count, duration, error count, and more. You can set up alarms to notify you of any issues and use CloudWatch Logs to debug your Lambda functions.
Caching
Improve the performance and reduce the load on your Lambda functions.
Enable caching in API Gateway.
API Gateway caching allows you to cache the responses of your API endpoints, reducing the number of calls to your Lambda functions. This can significantly improve the performance of your API, especially for endpoints that return static data. You can configure the cache size, TTL (time to live), and invalidation policies to suit your needs.
Error Handling
Provide meaningful error messages to your users and handle errors gracefully.
Implement error handling in your Lambda functions and API Gateway.
Use try-catch blocks in your Lambda functions to catch and handle errors. You can also configure API Gateway to return custom error responses based on the status code. For example, you can return a detailed error message for a 400 Bad Request or a 500 Internal Server Error.
Rate Limiting and Throttling
Protect your API from being overwhelmed by too many requests.
Use API Gateway’s built-in rate limiting and throttling features.
API Gateway allows you to set rate limits and burst limits for your API endpoints. This ensures that your API can handle a high volume of requests without being overwhelmed. You can configure these limits at the API stage or method level.
Versioning
Manage changes to your API and maintain backward compatibility.
Use API Gateway’s versioning features.
API Gateway allows you to create multiple versions of your API, each with its own endpoint. This allows you to make changes to your API without breaking existing clients. You can also use stages to manage different environments (e.g., development, staging, production) and deploy changes gradually.
Conclusion
Building scalable APIs with API Gateway and Lambda is like achieving serverless nirvana. You get all the benefits of a robust, scalable backend without the hassle of managing servers. By leveraging AWS’s powerful services, you can focus on writing code and delivering value to your users.
So, go forth and build amazing, scalable APIs! And remember, here at Binary Brain, we’re always here to help you navigate the complex world of web development with a smile and a chuckle. Happy coding!